Today with a pandemic life about covid 19, is very useful monitoring how is the new life of the people, is really healthy? are you sleep really good? are you walk enough?, that is a question that could response the healthy monitoring solution, how much calories, how much steps by day, how is the sleep, and also with this values create a historic data and in a base of treshold values you could create alarms and send it by email, telegram, slack, etc.
and for better visualization it was integrated with a grafana software that is incredible for create dashboard.
for the future is possible apply Machine learning for know how is the behavior of people that has the same age, or live in the same place, etc.
Grafana demo Visualization of all health data
how is working the visualization data with grafana software in a real time, in the visualization appears, steps, sleep heart rate and calories.
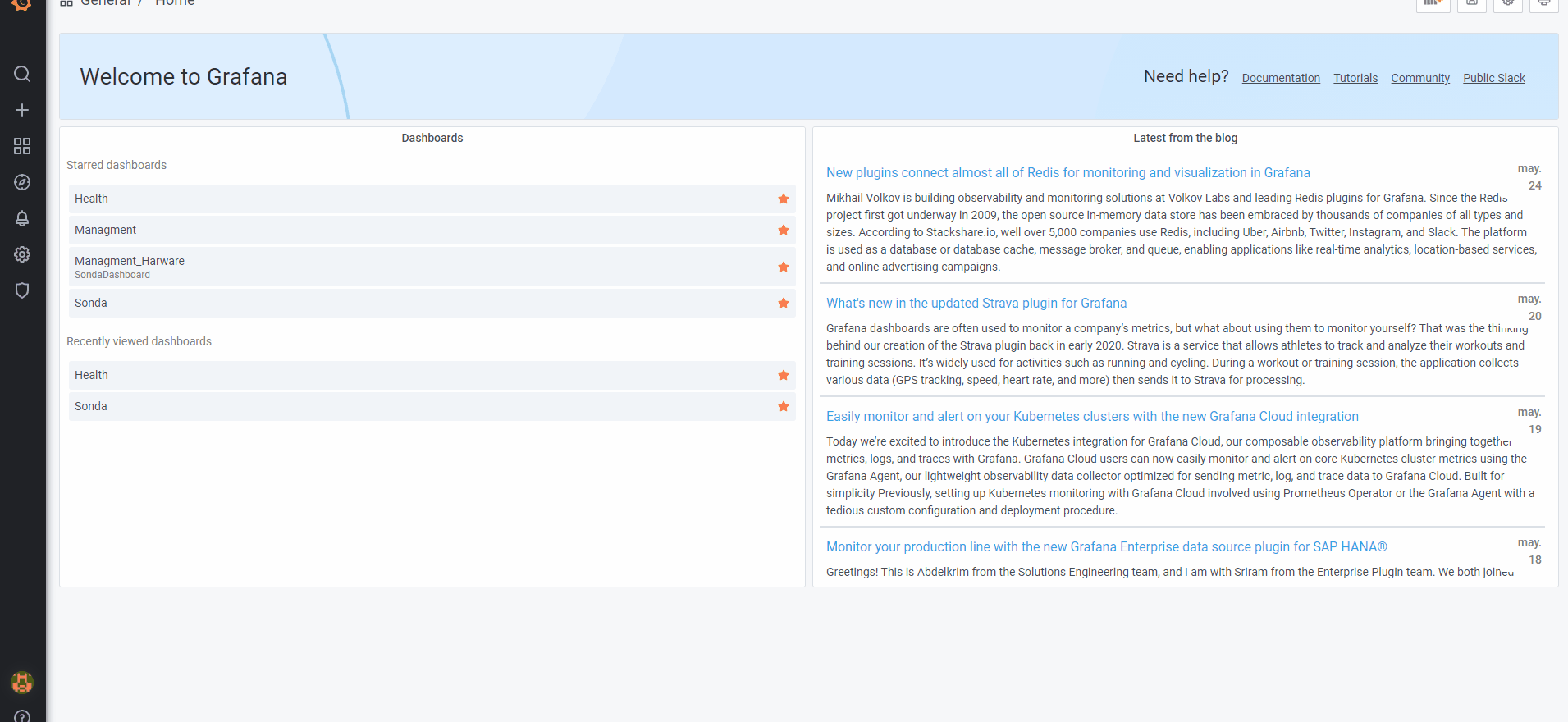
zabbix demo software with data from Garmin cloud
how is working the visualization of heart rate with zabbix software in a real time
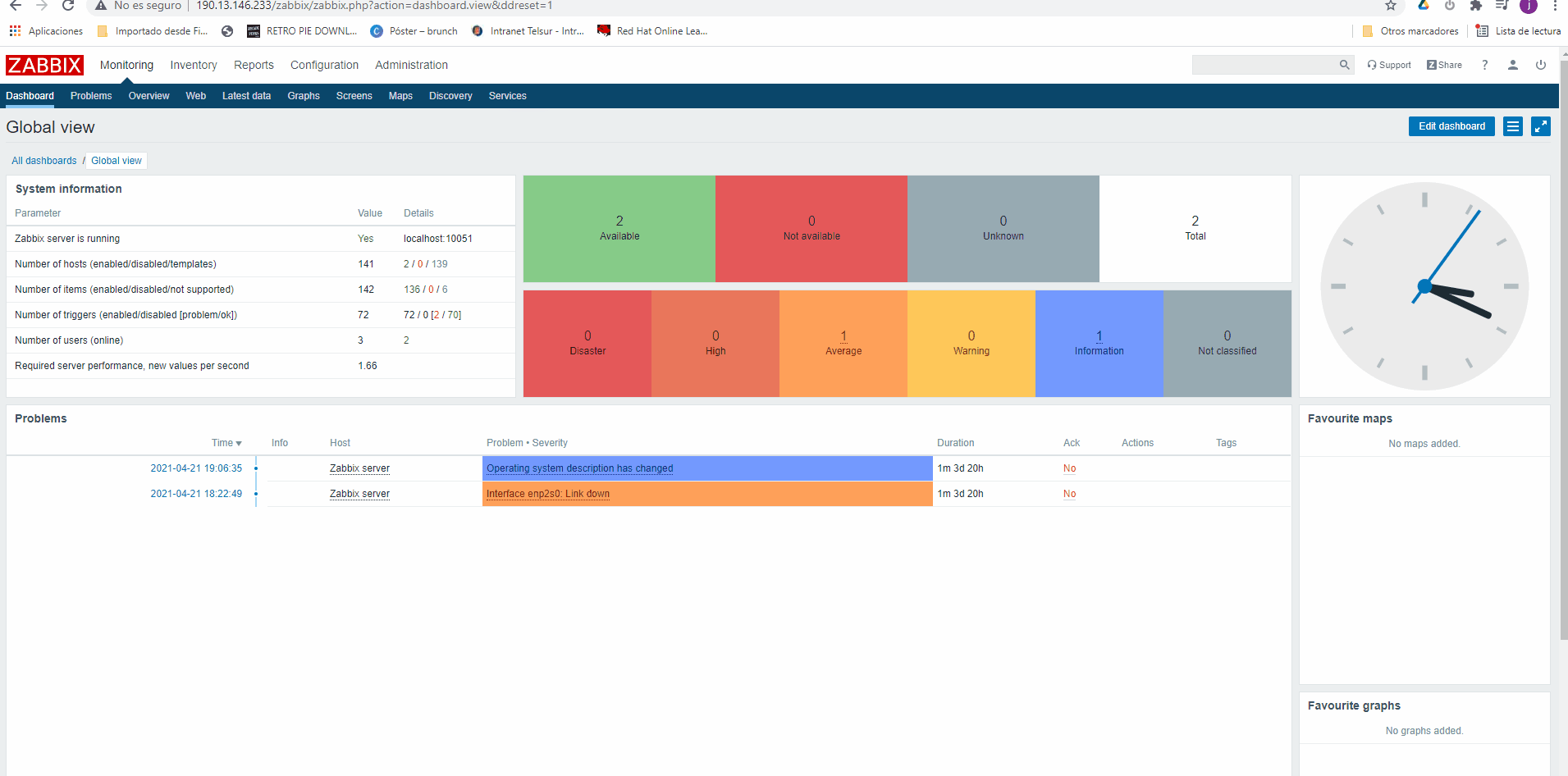
#!/usr/bin/env python3
import json
import re
import os
import time
import datetime
from garminconnect import (
Garmin,
GarminConnectConnectionError,
GarminConnectTooManyRequestsError,
GarminConnectAuthenticationError,
)
from datetime import date
"""
Enable debug logging
"""
import logging
logging.basicConfig(level=logging.INFO)
today = date.today()
print ("Start : %s" % time.ctime())
path = '/tmp/'
ipZabbix = '127.0.0.1'
"""
Initialize Garmin client with credentials
Only needed when your program is initialized
"""
YOUR_EMAIL='mail@gmail.com'
YOUR_PASSWORD='pass'
print("Garmin(email, password)")
print("----------------------------------------------------------------------------------------")
client = Garmin(YOUR_EMAIL, YOUR_PASSWORD)
try:
client = Garmin(YOUR_EMAIL, YOUR_PASSWORD)
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client init: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client init")
quit()
"""
Login to Garmin Connect portal
Only needed at start of your program
The library will try to relogin when session expires
"""
print("client.login()")
print("----------------------------------------------------------------------------------------")
try:
client.login()
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client login: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client login")
quit()
"""
Get heart rate data
"""
#print("client.get_heart_rates(%s)", today.isoformat())
print("----------------------------------------------------------------------------------------")
host = "GarminData"
key = "heartrate"
fileheart = 'insertZabbix.log'
logZabbix = open(path+fileheart,'w')
try:
heartRateToday = client.get_heart_rates(today.isoformat())
dictHeart = heartRateToday['heartRateValues']
#print(dictHeart)
count = 0
for timestamp, heart in dictHeart:
#print(timestamp, '-->', heart)
tiempo = str(timestamp)
lenvalue = len(str(tiempo))
newTime = tiempo[:lenvalue-3]
#print(timestamp)
#print(newTime)
#print(('datos procesados: %s')%(count))
count = count + 1
if heart:
logZabbix.write(('"%s" %s %s %s\n')%(host,key,newTime,heart))
logZabbix.close()
os.system(("/usr/bin/zabbix_sender -z %s -i %s -T") %(ipZabbix,path+fileheart))
time.sleep( 5 )
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client get heart rates data: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client get heart rates data")
quit()
"""
Get activity data
"""
#print("client.get_stats(%s)", today.isoformat())
print("client.get_stats(%s)", today.isoformat())
print("----------------------------------------------------------------------------------------")
try:
#print(client.get_stats(today.isoformat()))
datatStats = client.get_stats(today.isoformat())
totalKilocalories = datatStats['totalKilocalories']
activeKilocalories = datatStats['activeKilocalories']
bmrKilocalories = datatStats['bmrKilocalories']
totalSteps = datatStats['totalSteps']
totalDistanceMeters = datatStats['totalDistanceMeters']
calendarDate = datatStats['calendarDate']
minAvgHeartRate = datatStats['minAvgHeartRate']
maxAvgHeartRate = datatStats['maxAvgHeartRate']
dateTimestamp = int(time.mktime(datetime.datetime.strptime(calendarDate, "%Y-%m-%d").timetuple()))
stats = 'insertGet_stats.log'
logget_stats = open(path+stats,'w')
key = 'totalKilocalories'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,totalKilocalories))
key = 'activeKilocalories'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,activeKilocalories))
key = 'bmrKilocalories'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,bmrKilocalories))
key = 'totalSteps'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,totalSteps))
key = 'totalDistanceMeters'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,totalDistanceMeters))
key = 'minAvgHeartRate'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,minAvgHeartRate))
key = 'maxAvgHeartRate'
logget_stats.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,maxAvgHeartRate))
logget_stats.close()
os.system(("/usr/bin/zabbix_sender -z %s -i %s -T") %(ipZabbix,path+stats))
time.sleep( 5 )
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client get stats: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client get stats")
quit()
#heartRateToday = client.get_heart_rates(today.isoformat())
#heart_dict = json.loads(heartRateToday)
#print(heart_dict)
'''
try:
#print(client.get_heart_rates(today.isoformat()))
heartRateToday = client.get_heart_rates(today.isoformat())
heart_dict = json.loads(heartRateToday)
print(heart_dict)
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client get heart rates: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client get heart rates")
quit()
'''
"""
Get sleep data
"""
print("client.get_sleep_data(%s)", today.isoformat())
print("----------------------------------------------------------------------------------------")
try:
#print(client.get_sleep_data(today.isoformat()))
sleep = client.get_sleep_data(today.isoformat())
print('sleep data')
dictSleep = sleep['sleepMovement']
sleep = 'insertSleep.log'
logSleep = open(path+sleep,'w')
for data in dictSleep:
#print(data['startGMT'] ,' --> ',data['endGMT'] ,' : ' ,data['activityLevel'])
dateTimestamp = int(time.mktime(datetime.datetime.strptime(data['startGMT'], "%Y-%m-%dT%H:%M:%S.%f").timetuple()))
key = 'sleep'
logSleep.write(('"%s" %s %s %s\n')%(host,key,dateTimestamp,data['activityLevel']))
logSleep.close()
os.system(("/usr/bin/zabbix_sender -z %s -i %s -T") %(ipZabbix,path+sleep))
time.sleep( 5 )
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client get sleep data: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client get sleep data")
quit()
"""
Get steps data
"""
#print("client.get_steps_data\(%s\)", today.isoformat())
print("----------------------------------------------------------------------------------------")
try:
#print(client.get_steps_data(today.isoformat()))
print('Steps data')
except (
GarminConnectConnectionError,
GarminConnectAuthenticationError,
GarminConnectTooManyRequestsError,
) as err:
print("Error occurred during Garmin Connect Client get steps data: %s" % err)
quit()
except Exception: # pylint: disable=broad-except
print("Unknown error occurred during Garmin Connect Client get steps data")
quit()
print("ok")
#print ("End : %s" % time.ctime())
3 projects • 3 followers
I'm electronic engineer, apassionated for developer things that can make easy to people live.
Comments
Please log in or sign up to comment.