Please log in or create an account to get started.
Join the world’s best hardware engineers.
Create your account to explore thousands of projects, build your skills, and discover new products and technologies.
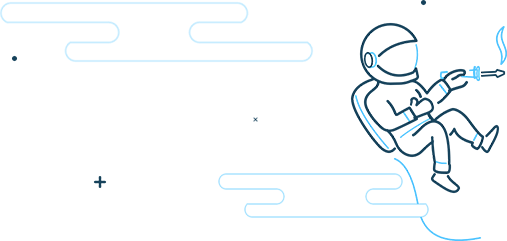
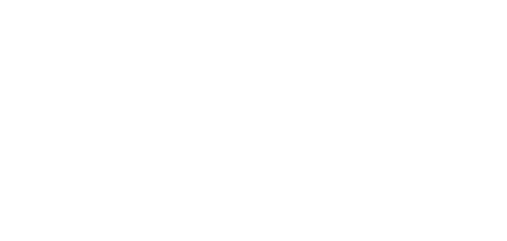
Create your account to explore thousands of projects, build your skills, and discover new products and technologies.