Hardware components | ||||||
![]() |
| × | 1 | |||
![]() |
| × | 16 | |||
![]() |
| × | 4 | |||
![]() |
| × | 8 | |||
| × | 1 | ||||
| × | 1 | ||||
Software apps and online services | ||||||
![]() |
| |||||
![]() |
| |||||
Hand tools and fabrication machines | ||||||
![]() |
| |||||
![]() |
|
The topper is the most important decoration of a Christmas tree. There are numerous types of toppers, but IoT toppers... Well that's a quite new thing and I built one. Here's how you can build one, too.
The shapeAt first you will need some cool decoration to work with. I bought a polystyrene star in a paper shop, but if you've got a 3D printer your possibilities are endless.
I painted the star yellow with a marker and dug holes for the LEDs with a needle. You can find where to dig the holes using the "IDs of the LEDs" picture in the Schematics section. Place the LEDs in the holes and start soldering them together based on the "circuit schematics" that I provided. Polystyrene is heat sensitive, so partly pull out the LED when you solder it and be fast. When it is required create bridges with insulating tapes.
My circuit diagram is possibly not optimal, so if you've got any better idea go with it. The wires next to the LEDs are connections to the + and - lines (4-4). At this point you've got to do two things: solder the wires onto the female headers bottom and hot glue them onto the back of the star. I soldered the positive lines to the top part and the negative lines to the bottom part of the headers.
You have to connect 220Ω resistors to either the + or - lines to complete the circuits. You can build them onto the star but I used external resistors. I soldered a couple of male headers and 4 resistors together and I got this:
This project uses Blynk. If you don't know Blynk click here for more info.
Download the Blynk app from the Google Play or Apple's App Store and login. Unfortunately Blynk gives you only 2000 energy (?) for free and a button costs 200 so you either buy some more energy or decrease the functionality of the star. A third option is you modify my project for example by using next/previous buttons instead of selecting the lighting modes directly. If you stay with option one or you've got more than 2000 energy, this is what you need to make to control the star in Blynk:
You will need 3 types of modules here, a Value Display ("Lighting mode", input: V0), a Slider ("Speed", output: V1, Range: 40-1, but you can change the range if you wish) and Buttons. All buttons are set to PUSH, their labels are their Lighting mode IDs (see the second picture on top of this text) and their names are:
- V2 - Simple on
- V3 - Flashing
- V4 - Fading
- V5 - Off
- V6 - Running in
- V7 - Running out
- V8 - Fading in
- V9 - Fading out
- V10 - Inverse fading in
- V11 - Inverse fading out
- V12 - Run around CW (CW = Clockwise)
- V13 - Run around CCW ( CCW = Counterclockwise)
- V14 - Fade around CW 1
- V15 - Fade around CCW 1
- V16 - Fade around CW 2
- V17 - Fade around CCW 2
When you create the Blynk project, you will get an authorization token. You will need that later when you upload the code into the MKR1000.
After that, all you need to do is connect the star to the Arduino MKR1000 like this:
- P4 line -> Arduino D2
- P3 line -> Arduino D3
- P2 line -> Arduino D4
- P1 line -> Arduino D5
- N4 line -> Arduino D6
- N3 line -> Arduino D7
- N2 line -> Arduino D8
- N1 line -> Arduino D9
Upload my code to your MKR1000 (fill the SSID, password and authorization variables with proper values. Auth is the string that I mentioned when you created the project in Blynk) and now all you have to do is put your topper on your Christmas tree and hide the wires.
I'll write some words about the code, too. The star display works like a Matrix display or a 2/3/4 digit 7 segment display, except it is in a star shape. My code handles the LEDs accordingly. The star has 4 groups of LEDs, and there are 4 LEDs in each group. The "plot" array in the code contains the 256 step long program for display update. When you push a button in Blynk, the proper Blynk function executes in the MCU that overwrites the plot array and thus it changes what the MKR1000 displays. This is a simple code, but it is also quite long, so feel free to ask, if something is not clear.
If you are here sometime around December in any year then I wish you a Merry Christmas and Happy New Year! If not then I just simply thank you for being interested in my project!
The circuit of the star display
negative lines. Solder the LEDs accordingly. Each line is connected to 4 LEDs. The numbers refer to the variables in the code/pins of the MCU. I think it's easy to understand, but if you have difficulties contact me and if it is required I will think of something else.
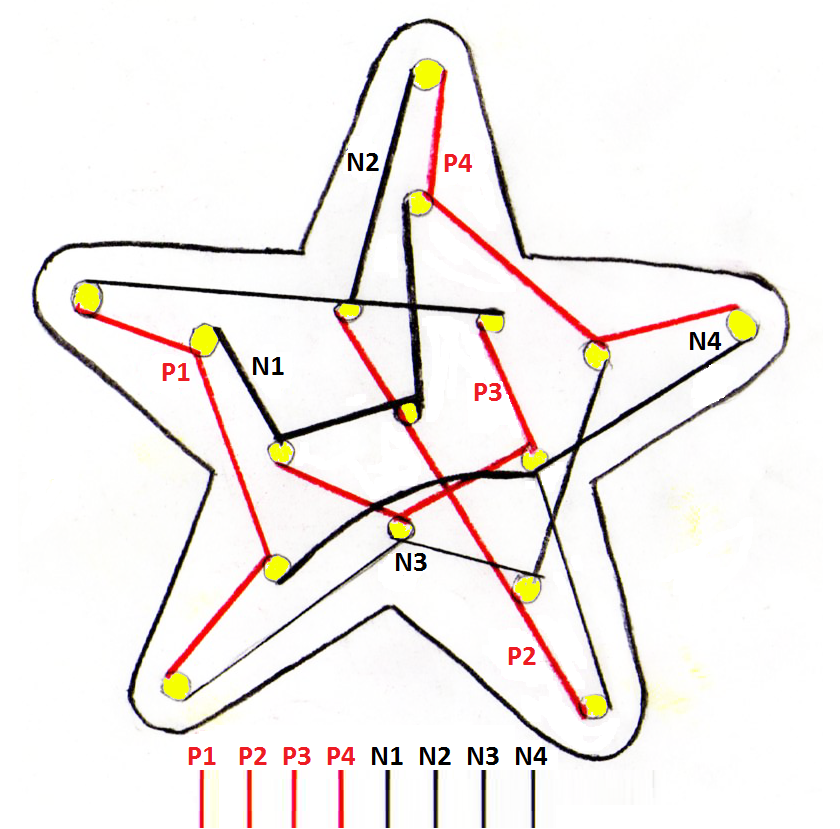
Star controller code
Arduino/**************************************************************
* This code controls a star shaped DIY display using an
* Arduino MKR1000 and Blynk. More info:
* https://www.hackster.io/Abysmal/iot-christmas-tree-topper-7ad7e8
*
* This project is made for "The Arduino Internet of Holiday Things"
* contest on hackster.io. More info:
* https://www.hackster.io/contests/arduino-holiday-contest
*
* author: Balázs Simon
*
**************************************************************/
#include <SPI.h>
#include <WiFi101.h>
#include <BlynkSimpleMKR1000.h>
#define P4 2
#define P3 3
#define P2 4
#define P1 5
#define N4 6
#define N3 7
#define N2 8
#define N1 9
#define PLOT_LENGTH 256
// You should get Auth Token in the Blynk App.
// Go to the Project Settings (nut icon).
char auth[] = "YourAuthToken";
// Your WiFi credentials.
// Set password to "" for open networks.
char ssid[] = "YourNetworkName";
char password[] = "YourPassword";
int lightingMode = 1;
int changeSpeed = 1;
char plot[16][PLOT_LENGTH];
int currentStep = 0;
unsigned long lastLEDUpdateTime = 0;
void setup() {
Blynk.begin(auth, ssid, password);
pinMode(P1, OUTPUT);
pinMode(P2, OUTPUT);
pinMode(P3, OUTPUT);
pinMode(P4, OUTPUT);
pinMode(N1, OUTPUT);
pinMode(N2, OUTPUT);
pinMode(N3, OUTPUT);
pinMode(N4, OUTPUT);
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 255;
}
}
}
void loop() {
//Star screen updating based on the "plot"
for (int i = 0; i < 256; i++) {
int j = 0;
digitalWrite(N4, HIGH);
plot[j++][currentStep] > i ? digitalWrite(P1, HIGH) : digitalWrite(P1, LOW);
plot[j++][currentStep] > i ? digitalWrite(P2, HIGH) : digitalWrite(P2, LOW);
plot[j++][currentStep] > i ? digitalWrite(P3, HIGH) : digitalWrite(P3, LOW);
plot[j++][currentStep] > i ? digitalWrite(P4, HIGH) : digitalWrite(P4, LOW);
digitalWrite(N1, LOW);
delayMicroseconds(20);
digitalWrite(N1, HIGH);
plot[j++][currentStep] > i ? digitalWrite(P1, HIGH) : digitalWrite(P1, LOW);
plot[j++][currentStep] > i ? digitalWrite(P2, HIGH) : digitalWrite(P2, LOW);
plot[j++][currentStep] > i ? digitalWrite(P3, HIGH) : digitalWrite(P3, LOW);
plot[j++][currentStep] > i ? digitalWrite(P4, HIGH) : digitalWrite(P4, LOW);
digitalWrite(N2, LOW);
delayMicroseconds(20);
digitalWrite(N2, HIGH);
plot[j++][currentStep] > i ? digitalWrite(P1, HIGH) : digitalWrite(P1, LOW);
plot[j++][currentStep] > i ? digitalWrite(P2, HIGH) : digitalWrite(P2, LOW);
plot[j++][currentStep] > i ? digitalWrite(P3, HIGH) : digitalWrite(P3, LOW);
plot[j++][currentStep] > i ? digitalWrite(P4, HIGH) : digitalWrite(P4, LOW);
digitalWrite(N3, LOW);
delayMicroseconds(20);
digitalWrite(N3, HIGH);
plot[j++][currentStep] > i ? digitalWrite(P1, HIGH) : digitalWrite(P1, LOW);
plot[j++][currentStep] > i ? digitalWrite(P2, HIGH) : digitalWrite(P2, LOW);
plot[j++][currentStep] > i ? digitalWrite(P3, HIGH) : digitalWrite(P3, LOW);
plot[j++][currentStep] > i ? digitalWrite(P4, HIGH) : digitalWrite(P4, LOW);
digitalWrite(N4, LOW);
delayMicroseconds(20);
}
if (millis() - lastLEDUpdateTime > changeSpeed) {
//Moving to the next image in the plot
lastLEDUpdateTime = millis();
currentStep++;
if (currentStep == PLOT_LENGTH) {
currentStep = 0;
}
}
Blynk.run();
}
//Speed slider
BLYNK_WRITE(V1)
{
changeSpeed = param.asInt();
}
//Creating "Simple on" plot
BLYNK_WRITE(V2)
{
if (lightingMode != 1) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 255;
}
}
lightingMode = 1;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Flashing" plot
BLYNK_WRITE(V3)
{
if (lightingMode != 2) {
for (int i = 0; i < PLOT_LENGTH / 2; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 255;
}
}
for (int i = PLOT_LENGTH / 2; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
}
lightingMode = 2;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fading" plot
BLYNK_WRITE(V4)
{
if (lightingMode != 3) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
if (i == 0) {
plot[j][i] = 255;
}
else if (i * 2 < PLOT_LENGTH) {
plot[j][i] = PLOT_LENGTH - 2 * i;
}
else {
plot[j][i] = 2 * i - PLOT_LENGTH;
}
}
}
lightingMode = 3;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Off" plot
BLYNK_WRITE(V5)
{
if (lightingMode != 4) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
}
lightingMode = 4;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Running in" plot
BLYNK_WRITE(V6)
{
if (lightingMode != 5) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 2) {
plot[0][i] = 255;
plot[2][i] = 255;
plot[7][i] = 255;
plot[8][i] = 255;
plot[11][i] = 255;
}
else {
plot[0][i] = 0;
plot[2][i] = 0;
plot[7][i] = 0;
plot[8][i] = 0;
plot[11][i] = 0;
}
if (i - 32 < PLOT_LENGTH / 2 && i >= 32) {
plot[3][i] = 255;
plot[4][i] = 255;
plot[6][i] = 255;
plot[12][i] = 255;
plot[15][i] = 255;
}
else {
plot[3][i] = 0;
plot[4][i] = 0;
plot[6][i] = 0;
plot[12][i] = 0;
plot[15][i] = 0;
}
if (i - 64 < PLOT_LENGTH / 2 && i >= 64) {
plot[1][i] = 255;
plot[5][i] = 255;
plot[9][i] = 255;
plot[10][i] = 255;
plot[13][i] = 255;
}
else {
plot[1][i] = 0;
plot[5][i] = 0;
plot[9][i] = 0;
plot[10][i] = 0;
plot[13][i] = 0;
}
if (i - 96 < PLOT_LENGTH / 2 && i >= 96) {
plot[14][i] = 255;
}
else {
plot[14][i] = 0;
}
}
lightingMode = 5;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Running out" plot
BLYNK_WRITE(V7)
{
if (lightingMode != 6) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 2) {
plot[14][i] = 255;
}
else {
plot[14][i] = 0;
}
if (i - 32 < PLOT_LENGTH / 2 && i >= 32) {
plot[1][i] = 255;
plot[5][i] = 255;
plot[9][i] = 255;
plot[10][i] = 255;
plot[13][i] = 255;
}
else {
plot[1][i] = 0;
plot[5][i] = 0;
plot[9][i] = 0;
plot[10][i] = 0;
plot[13][i] = 0;
}
if (i - 64 < PLOT_LENGTH / 2 && i >= 64) {
plot[3][i] = 255;
plot[4][i] = 255;
plot[6][i] = 255;
plot[12][i] = 255;
plot[15][i] = 255;
}
else {
plot[3][i] = 0;
plot[4][i] = 0;
plot[6][i] = 0;
plot[12][i] = 0;
plot[15][i] = 0;
}
if (i - 96 < PLOT_LENGTH / 2 && i >= 96) {
plot[0][i] = 255;
plot[2][i] = 255;
plot[7][i] = 255;
plot[8][i] = 255;
plot[11][i] = 255;
}
else {
plot[0][i] = 0;
plot[2][i] = 0;
plot[7][i] = 0;
plot[8][i] = 0;
plot[11][i] = 0;
}
}
lightingMode = 6;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fading in" plot
BLYNK_WRITE(V8)
{
if (lightingMode != 7) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 4) {
plot[0][i] = 4 * i;
plot[2][i] = 4 * i;
plot[7][i] = 4 * i;
plot[8][i] = 4 * i;
plot[11][i] = 4 * i;
}
else if (i - 64 < PLOT_LENGTH / 4) {
plot[0][i] = 255 - 4 * (i - 64);
plot[2][i] = 255 - 4 * (i - 64);
plot[7][i] = 255 - 4 * (i - 64);
plot[8][i] = 255 - 4 * (i - 64);
plot[11][i] = 255 - 4 * (i - 64);
}
else {
plot[0][i] = 0;
plot[2][i] = 0;
plot[7][i] = 0;
plot[8][i] = 0;
plot[11][i] = 0;
}
if (i - 32 < PLOT_LENGTH / 4 && i >= 32) {
plot[3][i] = 4 * (i - 32);
plot[4][i] = 4 * (i - 32);
plot[6][i] = 4 * (i - 32);
plot[12][i] = 4 * (i - 32);
plot[15][i] = 4 * (i - 32);
}
else if (i - 96 < PLOT_LENGTH / 4 && i >= 32) {
plot[3][i] = 255 - 4 * (i - 96);
plot[4][i] = 255 - 4 * (i - 96);
plot[6][i] = 255 - 4 * (i - 96);
plot[12][i] = 255 - 4 * (i - 96);
plot[15][i] = 255 - 4 * (i - 96);
}
else {
plot[3][i] = 0;
plot[4][i] = 0;
plot[6][i] = 0;
plot[12][i] = 0;
plot[15][i] = 0;
}
if (i - 64 < PLOT_LENGTH / 4 && i > 64) {
plot[1][i] = 4 * (i - 64);
plot[5][i] = 4 * (i - 64);
plot[9][i] = 4 * (i - 64);
plot[10][i] = 4 * (i - 64);
plot[13][i] = 4 * (i - 64);
}
else if (i - 128 < PLOT_LENGTH / 4 && i > 64) {
plot[1][i] = 255 - 4 * (i - 128);
plot[5][i] = 255 - 4 * (i - 128);
plot[9][i] = 255 - 4 * (i - 128);
plot[10][i] = 255 - 4 * (i - 128);
plot[13][i] = 255 - 4 * (i - 128);
}
else {
plot[1][i] = 0;
plot[5][i] = 0;
plot[9][i] = 0;
plot[10][i] = 0;
plot[13][i] = 0;
}
if (i - 96 < PLOT_LENGTH / 4 && i >= 96) {
plot[14][i] = 4 * (i - 96);
}
else if (i - 160 < PLOT_LENGTH / 4 && i >= 96) {
plot[14][i] = 255 - 4 * (i - 160);
}
else {
plot[14][i] = 0;
}
}
lightingMode = 7;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fading out" plot
BLYNK_WRITE(V9)
{
if (lightingMode != 8) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 4) {
plot[14][i] = 4 * i;
}
else if (i - 64 < PLOT_LENGTH / 4) {
plot[14][i] = 255 - 4 * (i - 64);
}
else {
plot[14][i] = 0;
}
if (i - 32 < PLOT_LENGTH / 4 && i >= 32) {
plot[1][i] = 4 * (i - 32);
plot[5][i] = 4 * (i - 32);
plot[9][i] = 4 * (i - 32);
plot[10][i] = 4 * (i - 32);
plot[13][i] = 4 * (i - 32);
}
else if (i - 96 < PLOT_LENGTH / 4 && i >= 32) {
plot[1][i] = 255 - 4 * (i - 96);
plot[5][i] = 255 - 4 * (i - 96);
plot[9][i] = 255 - 4 * (i - 96);
plot[10][i] = 255 - 4 * (i - 96);
plot[13][i] = 255 - 4 * (i - 96);
}
else {
plot[1][i] = 0;
plot[5][i] = 0;
plot[9][i] = 0;
plot[10][i] = 0;
plot[13][i] = 0;
}
if (i - 64 < PLOT_LENGTH / 4 && i >= 64) {
plot[3][i] = 4 * (i - 64);
plot[4][i] = 4 * (i - 64);
plot[6][i] = 4 * (i - 64);
plot[12][i] = 4 * (i - 64);
plot[15][i] = 4 * (i - 64);
}
else if (i - 128 < PLOT_LENGTH / 4 && i >= 64) {
plot[3][i] = 255 - 4 * (i - 128);
plot[4][i] = 255 - 4 * (i - 128);
plot[6][i] = 255 - 4 * (i - 128);
plot[12][i] = 255 - 4 * (i - 128);
plot[15][i] = 255 - 4 * (i - 128);
}
else {
plot[3][i] = 0;
plot[4][i] = 0;
plot[6][i] = 0;
plot[12][i] = 0;
plot[15][i] = 0;
}
if (i - 96 < PLOT_LENGTH / 4 && i >= 96) {
plot[0][i] = 4 * (i - 96);
plot[2][i] = 4 * (i - 96);
plot[7][i] = 4 * (i - 96);
plot[8][i] = 4 * (i - 96);
plot[11][i] = 4 * (i - 96);
}
else if (i - 160 < PLOT_LENGTH / 4 && i >= 96) {
plot[0][i] = 255 - 4 * (i - 160);
plot[2][i] = 255 - 4 * (i - 160);
plot[7][i] = 255 - 4 * (i - 160);
plot[8][i] = 255 - 4 * (i - 160);
plot[11][i] = 255 - 4 * (i - 160);
}
else {
plot[0][i] = 0;
plot[2][i] = 0;
plot[7][i] = 0;
plot[8][i] = 0;
plot[11][i] = 0;
}
}
lightingMode = 8;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Inverse fading in" plot
BLYNK_WRITE(V10)
{
if (lightingMode != 9) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 4) {
plot[0][i] = 255 - 4 * i;
plot[2][i] = 255 - 4 * i;
plot[7][i] = 255 - 4 * i;
plot[8][i] = 255 - 4 * i;
plot[11][i] = 255 - 4 * i;
}
else if (i - 64 < PLOT_LENGTH / 4) {
plot[0][i] = 4 * (i - 64);
plot[2][i] = 4 * (i - 64);
plot[7][i] = 4 * (i - 64);
plot[8][i] = 4 * (i - 64);
plot[11][i] = 4 * (i - 64);
}
else {
plot[0][i] = 255;
plot[2][i] = 255;
plot[7][i] = 255;
plot[8][i] = 255;
plot[11][i] = 255;
}
if (i - 32 < PLOT_LENGTH / 4 && i >= 32) {
plot[3][i] = 255 - 4 * (i - 32);
plot[4][i] = 255 - 4 * (i - 32);
plot[6][i] = 255 - 4 * (i - 32);
plot[12][i] = 255 - 4 * (i - 32);
plot[15][i] = 255 - 4 * (i - 32);
}
else if (i - 96 < PLOT_LENGTH / 4 && i >= 32) {
plot[3][i] = 4 * (i - 96);
plot[4][i] = 4 * (i - 96);
plot[6][i] = 4 * (i - 96);
plot[12][i] = 4 * (i - 96);
plot[15][i] = 4 * (i - 96);
}
else {
plot[3][i] = 255;
plot[4][i] = 255;
plot[6][i] = 255;
plot[12][i] = 255;
plot[15][i] = 255;
}
if (i - 64 < PLOT_LENGTH / 4 && i >= 64) {
plot[1][i] = 255 - 4 * (i - 64);
plot[5][i] = 255 - 4 * (i - 64);
plot[9][i] = 255 - 4 * (i - 64);
plot[10][i] = 255 - 4 * (i - 64);
plot[13][i] = 255 - 4 * (i - 64);
}
else if (i - 128 < PLOT_LENGTH / 4 && i >= 64) {
plot[1][i] = 4 * (i - 128);
plot[5][i] = 4 * (i - 128);
plot[9][i] = 4 * (i - 128);
plot[10][i] = 4 * (i - 128);
plot[13][i] = 4 * (i - 128);
}
else {
plot[1][i] = 255;
plot[5][i] = 255;
plot[9][i] = 255;
plot[10][i] = 255;
plot[13][i] = 255;
}
if (i - 96 < PLOT_LENGTH / 4 && i >= 96) {
plot[14][i] = 255 - 4 * (i - 96);
}
else if (i - 160 < PLOT_LENGTH / 4 && i >= 96) {
plot[14][i] = 4 * (i - 160);
}
else {
plot[14][i] = 255;
}
}
lightingMode = 9;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Inverse fading out" plot
BLYNK_WRITE(V11)
{
if (lightingMode != 10) {
for (int i = 0; i < PLOT_LENGTH; i++) {
if (i < PLOT_LENGTH / 4) {
plot[14][i] = 255 - 4 * i;
}
else if (i - 64 < PLOT_LENGTH / 4) {
plot[14][i] = 4 * (i - 64);
}
else {
plot[14][i] = 255;
}
if (i - 32 < PLOT_LENGTH / 4 && i >= 32) {
plot[1][i] = 255 - 4 * (i - 32);
plot[5][i] = 255 - 4 * (i - 32);
plot[9][i] = 255 - 4 * (i - 32);
plot[10][i] = 255 - 4 * (i - 32);
plot[13][i] = 255 - 4 * (i - 32);
}
else if (i - 96 < PLOT_LENGTH / 4 && i >= 32) {
plot[1][i] = 4 * (i - 96);
plot[5][i] = 4 * (i - 96);
plot[9][i] = 4 * (i - 96);
plot[10][i] = 4 * (i - 96);
plot[13][i] = 4 * (i - 96);
}
else {
plot[1][i] = 255;
plot[5][i] = 255;
plot[9][i] = 255;
plot[10][i] = 255;
plot[13][i] = 255;
}
if (i - 64 < PLOT_LENGTH / 4 && i >= 64) {
plot[3][i] = 255 - 4 * (i - 64);
plot[4][i] = 255 - 4 * (i - 64);
plot[6][i] = 255 - 4 * (i - 64);
plot[12][i] = 255 - 4 * (i - 64);
plot[15][i] = 255 - 4 * (i - 64);
}
else if (i - 128 < PLOT_LENGTH / 4 && i >= 64) {
plot[3][i] = 4 * (i - 128);
plot[4][i] = 4 * (i - 128);
plot[6][i] = 4 * (i - 128);
plot[12][i] = 4 * (i - 128);
plot[15][i] = 4 * (i - 128);
}
else {
plot[3][i] = 255;
plot[4][i] = 255;
plot[6][i] = 255;
plot[12][i] = 255;
plot[15][i] = 255;
}
if (i - 96 < PLOT_LENGTH / 4 && i >= 96) {
plot[0][i] = 255 - 4 * (i - 96);
plot[2][i] = 255 - 4 * (i - 96);
plot[7][i] = 255 - 4 * (i - 96);
plot[8][i] = 255 - 4 * (i - 96);
plot[11][i] = 255 - 4 * (i - 96);
}
else if (i - 160 < PLOT_LENGTH / 4 && i >= 96) {
plot[0][i] = 4 * (i - 160);
plot[2][i] = 4 * (i - 160);
plot[7][i] = 4 * (i - 160);
plot[8][i] = 4 * (i - 160);
plot[11][i] = 4 * (i - 160);
}
else {
plot[0][i] = 255;
plot[2][i] = 255;
plot[7][i] = 255;
plot[8][i] = 255;
plot[11][i] = 255;
}
}
lightingMode = 10;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Run around CW" plot
BLYNK_WRITE(V12)
{
if (lightingMode != 11) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
plot[11][i] = 255;
plot[8][i] = 255;
plot[7][i] = 255;
plot[0][i] = 255;
plot[2][i] = 255;
plot[14][i] = 255;
if (i < 25) {
plot[6][i] = 255;
plot[10][i] = 255;
}
else if (i >= 25 && i < 51) {
plot[1][i] = 255;
plot[15][i] = 255;
}
else if (i >= 51 && i < 76) {
plot[4][i] = 255;
plot[13][i] = 255;
}
else if (i >= 76 && i < 102) {
plot[3][i] = 255;
plot[9][i] = 255;
}
else if (i >= 102 && i < 127) {
plot[5][i] = 255;
plot[12][i] = 255;
}
else if (i >= 127 && i < 153) {
plot[6][i] = 255;
plot[10][i] = 255;
}
else if (i >= 153 && i < 178) {
plot[1][i] = 255;
plot[15][i] = 255;
}
else if (i >= 178 && i < 204) {
plot[4][i] = 255;
plot[13][i] = 255;
}
else if (i >= 204 && i <= 229) {
plot[3][i] = 255;
plot[9][i] = 255;
}
else if (i >= 229) {
plot[5][i] = 255;
plot[12][i] = 255;
}
}
lightingMode = 11;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Run around CCW" plot
BLYNK_WRITE(V13)
{
if (lightingMode != 12) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
plot[11][i] = 255;
plot[8][i] = 255;
plot[7][i] = 255;
plot[0][i] = 255;
plot[2][i] = 255;
plot[14][i] = 255;
if (i < 25) {
plot[5][i] = 255;
plot[12][i] = 255;
}
else if (i >= 25 && i < 51) {
plot[3][i] = 255;
plot[9][i] = 255;
}
else if (i >= 51 && i < 76) {
plot[4][i] = 255;
plot[13][i] = 255;
}
else if (i >= 76 && i < 102) {
plot[1][i] = 255;
plot[15][i] = 255;
}
else if (i >= 102 && i < 127) {
plot[6][i] = 255;
plot[10][i] = 255;
}
else if (i >= 127 && i < 153) {
plot[5][i] = 255;
plot[12][i] = 255;
}
else if (i >= 153 && i < 178) {
plot[3][i] = 255;
plot[9][i] = 255;
}
else if (i >= 178 && i < 204) {
plot[4][i] = 255;
plot[13][i] = 255;
}
else if (i >= 204 && i <= 229) {
plot[1][i] = 255;
plot[15][i] = 255;
}
else if (i >= 229) {
plot[6][i] = 255;
plot[10][i] = 255;
}
}
lightingMode = 12;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fade around CW 1" plot
BLYNK_WRITE(V14)
{
if (lightingMode != 13) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
plot[14][i] = 255;
if (i < 25) {
plot[10][i] = 255 - i * 10;
plot[15][i] = i * 10;
plot[11][i] = plot[15][i];
plot[1][i] = plot[15][i];
}
else if (i >= 25 && i < 51) {
plot[15][i] = 255 - (i - 25) * 10;
plot[11][i] = plot[15][i];
plot[1][i] = plot[15][i];
plot[13][i] = (i - 25) * 10;
}
else if (i >= 51 && i < 76) {
plot[13][i] = 255 - (i - 51) * 10;
plot[9][i] = (i - 51) * 10;
plot[7][i] = plot[9][i];
plot[3][i] = plot[9][i];
}
else if (i >= 76 && i < 102) {
plot[9][i] = 255 - (i - 76) * 10;
plot[7][i] = plot[9][i];
plot[3][i] = plot[9][i];
plot[5][i] = (i - 76) * 10;
}
else if (i >= 102 && i < 127) {
plot[5][i] = 255 - (i - 102) * 10;
plot[10][i] = (i - 102) * 10;
plot[6][i] = plot[10][i];
plot[2][i] = plot[10][i];
}
else if (i >= 127 && i < 153) {
plot[10][i] = 255 - (i - 127) * 10;
plot[6][i] = plot[10][i];
plot[2][i] = plot[10][i];
plot[1][i] = (i - 127) * 10;
}
else if (i >= 153 && i < 178) {
plot[1][i] = 255 - (i - 153) * 10;
plot[13][i] = (i - 153) * 10;
plot[4][i] = plot[13][i];
plot[0][i] = plot[13][i];
}
else if (i >= 178 && i < 204) {
plot[13][i] = 255 - (i - 178) * 10;
plot[4][i] = plot[13][i];
plot[9][i] = plot[13][i];
plot[9][i] = (i - 178) * 10;
}
else if (i >= 204 && i <= 229) {
plot[9][i] = 255 - (i - 204) * 10;
plot[12][i] = (i - 204) * 10;
plot[8][i] = plot[12][i];
plot[5][i] = plot[12][i];
}
else if (i >= 229 && i < 255) {
plot[12][i] = 255 - (i - 229) * 10;
plot[8][i] = plot[12][i];
plot[5][i] = plot[12][i];
plot[10][i] = (i - 229) * 10;
}
else{
plot[12][i] = 0;
plot[8][i] = plot[1][i];
plot[5][i] = plot[1][i];
plot[10][i] = 255;
}
}
lightingMode = 13;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fade around CCW 1" plot
BLYNK_WRITE(V15)
{
if (lightingMode != 14) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 0;
}
plot[14][i] = 255;
if (i < 25) {
plot[10][i] = 255 - i * 10;
plot[5][i] = i * 10;
plot[8][i] = plot[5][i];
plot[12][i] = plot[5][i];
}
else if (i >= 25 && i < 51) {
plot[5][i] = 255 - (i - 25) * 10;
plot[8][i] = plot[5][i];
plot[12][i] = plot[5][i];
plot[9][i] = (i - 25) * 10;
}
else if (i >= 51 && i < 76) {
plot[9][i] = 255 - (i - 51) * 10;
plot[0][i] = (i - 51) * 10;
plot[4][i] = plot[0][i];
plot[13][i] = plot[0][i];
}
else if (i >= 76 && i < 102) {
plot[0][i] = 255 - (i - 76) * 10;
plot[4][i] = plot[0][i];
plot[13][i] = plot[0][i];
plot[1][i] = (i - 76) * 10;
}
else if (i >= 102 && i < 127) {
plot[1][i] = 255 - (i - 102) * 10;
plot[2][i] = (i - 102) * 10;
plot[6][i] = plot[2][i];
plot[10][i] = plot[2][i];
}
else if (i >= 127 && i < 153) {
plot[2][i] = 255 - (i - 127) * 10;
plot[6][i] = plot[2][i];
plot[10][i] = plot[2][i];
plot[5][i] = (i - 127) * 10;
}
else if (i >= 153 && i < 178) {
plot[5][i] = 255 - (i - 153) * 10;
plot[3][i] = (i - 153) * 10;
plot[7][i] = plot[3][i];
plot[9][i] = plot[3][i];
}
else if (i >= 178 && i < 204) {
plot[3][i] = 255 - (i - 178) * 10;
plot[7][i] = plot[3][i];
plot[9][i] = plot[3][i];
plot[13][i] = (i - 178) * 10;
}
else if (i >= 204 && i <= 229) {
plot[13][i] = 255 - (i - 204) * 10;
plot[1][i] = (i - 204) * 10;
plot[11][i] = plot[1][i];
plot[15][i] = plot[1][i];
}
else if (i >= 229 && i < 255) {
plot[1][i] = 255 - (i - 229) * 10;
plot[11][i] = plot[1][i];
plot[15][i] = plot[1][i];
plot[10][i] = (i - 229) * 10;
}
else{
plot[1][i] = 0;
plot[11][i] = plot[1][i];
plot[15][i] = plot[1][i];
plot[10][i] = 255;
}
}
lightingMode = 14;
Blynk.virtualWrite(V1, changeSpeed);
Blynk.virtualWrite(V0, lightingMode);
}
}
//Creating "Fade around CW 2" plot
BLYNK_WRITE(V16)
{
if (lightingMode != 15) {
for (int i = 0; i < PLOT_LENGTH; i++) {
for (int j = 0; j < 16; j++) {
plot[j][i] = 255;
}
plot[14][i] = 0;
if (i < 25) {
plot[10][i] = i * 10;
plot[15][i] = 255 - i * 10;
plot[11][i] = plot[15][i];
plot[1][i] = plot[15][i];
}
else if (i >= 25 && i < 51) {
plot[15][i] = (i - 25) * 10;
plot[11][i] = plot[15][i];
plot[1][i] = plot[15][i];
plot[13][i] = 255 - (i - 25) * 10;
}
else if (i >= 51 && i < 76) {
plot[13][i] = (i - 51) * 10;
plot[9][i] = 255 - (i - 51) * 10;
plot[7][i] = plot[9][i];
plot[3][i] = plot[9][i];
}
else if (i >= 76 && i < 102) {
plot[9][i] = (i - 76) * 10;
...
This file has been truncated, please download it to see its full contents.
Comments
Please log in or sign up to comment.