Hardware components | ||||||
![]() |
| × | 1 | |||
| × | 1 | ||||
![]() |
| × | 3 | |||
Software apps and online services | ||||||
![]() |
|
This is a very easy project in wich I will show you how to first set up, and decode your remote. Afterwards you can always visit my other project if you want to get a little more advanced on this!
Schematics
Even though the IR reciever in the diagram is not the one I'm using, it's the only one I found in Fritzing, i'm using a VS1838
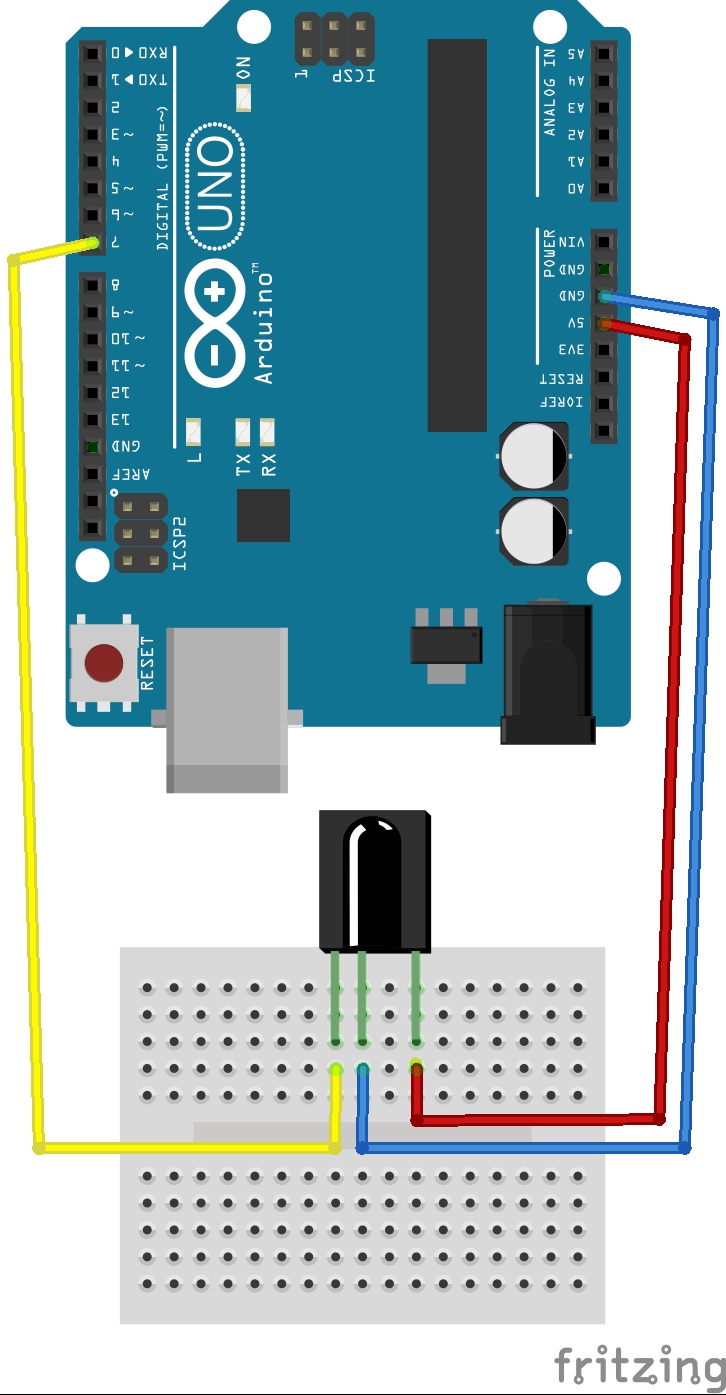
First Part
ArduinoAfter uploading this to the arduino, you should open the serial monitor (Tools), and press any button in the remote, (this should give you something like this "FD08F7"). After doing this with every button, we can get to the next part.
#include <IRremote.h>
const int RECV_PIN = 7;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup(){
Serial.begin(9600);
irrecv.enableIRIn();
}
void loop(){
if (irrecv.decode(&results)){
Serial.println(results.value, HEX);
irrecv.resume();
}
}
Second Part
ArduinoHere, You will be able to replace my remote values with yours to make it easier to see wich button you are pressing, and more organized for future projects.
Note: Before adding your HEX code, you will have to add a "0x" just as it is done in row 20, 23, etc
After doing this and uploading this code, instead of getting HEX values in your serial monitor, you should get what button you pressed
Note: Before adding your HEX code, you will have to add a "0x" just as it is done in row 20, 23, etc
After doing this and uploading this code, instead of getting HEX values in your serial monitor, you should get what button you pressed
#include <IRremote.h>
const int RECV_PIN = 7;
IRrecv irrecv(RECV_PIN);
decode_results results;
unsigned long key_value = 0;
void setup(){
Serial.begin(9600);
irrecv.enableIRIn();
}
void loop(){
if (irrecv.decode(&results)){
if (results.value == 0XFFFFFFFF)
results.value = key_value;
switch(results.value){
case 0xFD00FF:
Serial.println("ON / OFF");
break;
case 0xFD807F:
Serial.println("VOL+");
break;
case 0xFD40BF:
Serial.println("FUNC / STOP");
break;
case 0xFD20DF:
Serial.println("|<<");
break;
case 0xFDA05F:
Serial.println(">||");
break ;
case 0xFD609F:
Serial.println(">>|");
break ;
case 0xFD10EF:
Serial.println("");
break ;
case 0xFD906F:
Serial.println("VOL-");
break ;
case 0xFD50AF:
Serial.println("");
break ;
case 0xFD30CF:
Serial.println("0");
break ;
case 0xFDB04F:
Serial.println("EQ");
break ;
case 0xFD708F:
Serial.println("ST / REPT");
break ;
case 0xFD08F7:
Serial.println("1");
break ;
case 0xFD8877:
Serial.println("2");
break ;
case 0xFD48B7:
Serial.println("3");
break ;
case 0xFD28D7:
Serial.println("4");
break ;
case 0xFDA857:
Serial.println("5");
break ;
case 0xFD6897:
Serial.println("6");
break ;
case 0xFD18E7:
Serial.println("7");
break ;
case 0xFD9867:
Serial.println("8");
break ;
case 0xFD58A7:
Serial.println("9");
break ;
}
key_value = results.value;
irrecv.resume();
}
}
Comments
Please log in or sign up to comment.