Hardware components | ||||||
![]() |
| × | 2 | |||
![]() |
| × | 2 | |||
![]() |
| × | 1 | |||
| × | 5 | ||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
Software apps and online services | ||||||
| ||||||
![]() |
|
Generally, we need a right time to invest in any sort of cryptocurrencies we like but since most of us are not full time cryptoinvestors and the value of currency changes every second we could not figure best time to invest in it. and time goes on.. so to solve this problem I planned to build a system using BolT IoT so that it automatically notifies us when there is a right time to invest.
Bolt IoT has a very easy interface so that we can quickly build our product.
So let's get started...
Hardware connections-1
1. Connect positive end (i.e longer leg)of two led's to resistors and connect other end of resistors to pin 2 and 3 respectively in bolt wifi module.
2.negative end of the led's to ground in bolt wifi module
2.negative end of the led's to ground in bolt wifi module
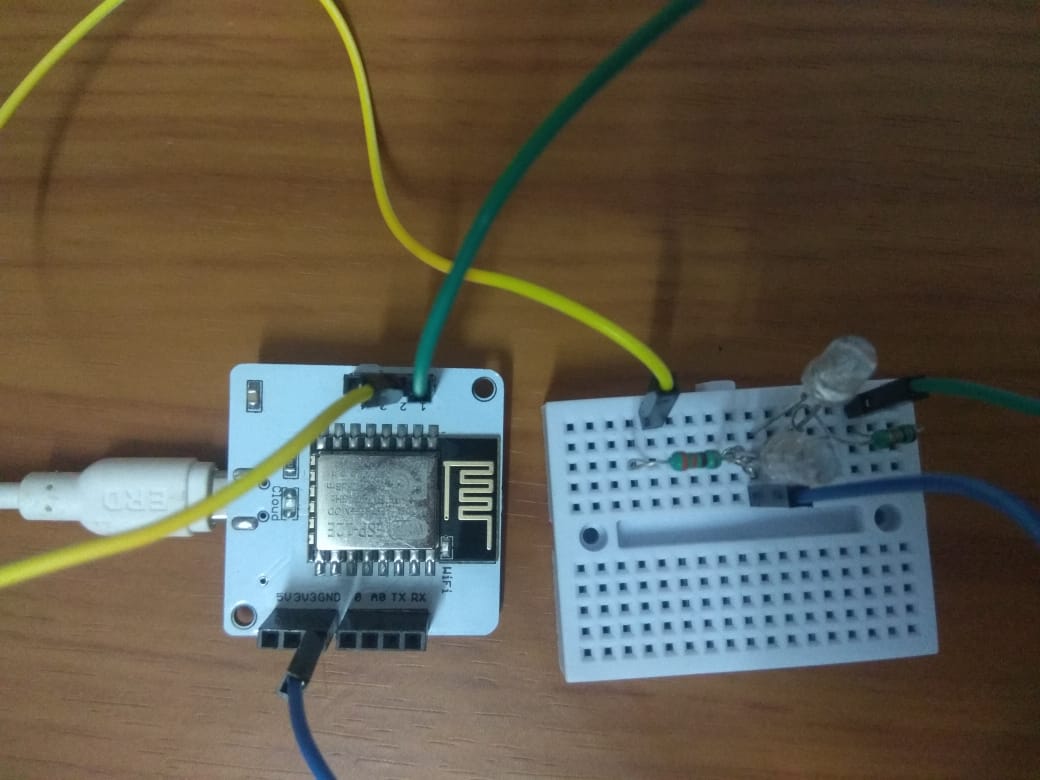
Hardware connections-2
1.Now,connect buzzer negative pin(i.e short leg) to ground pin .
2. Connect positive pin of buzzer to 0 pin of bolt wifi module.
This completes circuit.
3.connect bolt wifi module through USB cable and power on circuit.
4.connect to the bolt cloud
2. Connect positive pin of buzzer to 0 pin of bolt wifi module.
This completes circuit.
3.connect bolt wifi module through USB cable and power on circuit.
4.connect to the bolt cloud
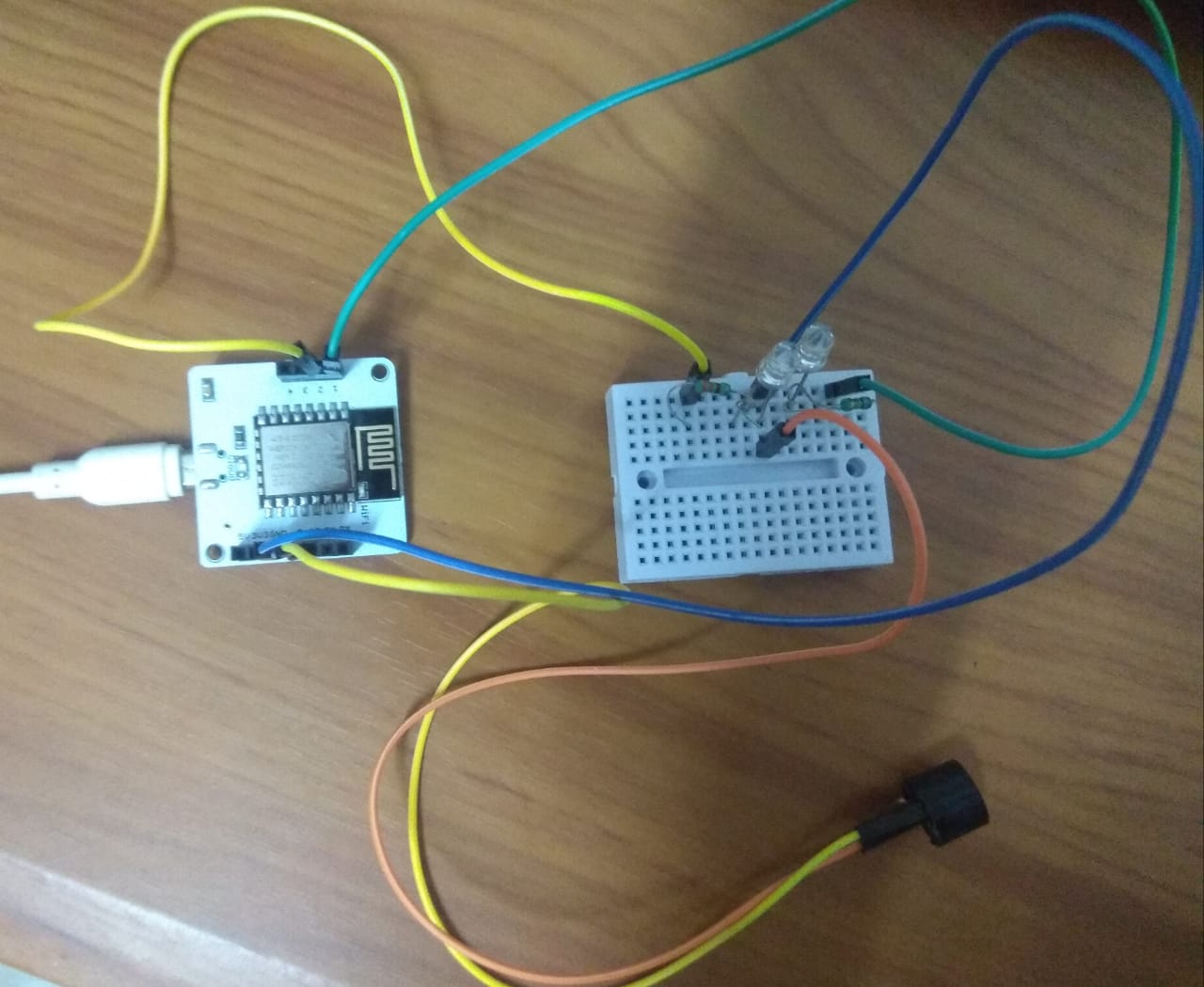
Getting all credentials
From bolt cloud we get api key and device id which are needed in conf.py file to connect to bolt cloud
_KLHSL6wASc.png)
Twilio connection
1. Needed to create an account in twilio and then from dash board we get accound sid,auth token,number which are needed in conf.py file to send notification.
_li_3OUv1BNdQ3.jpg)
currency_alert
PythonIn this code we get the current price of bitcoin and ethereum and sends us a message and notifies through buzzer and led's if both are available for us to invest at their best price or else notifies if any one of them are available .
import conf
import cryptocompare
from boltiot import Sms, Bolt
import json, time
bolt = Bolt(conf.API_KEY, conf.DEVICE_ID)#connecting to bolt cloud
sms = Sms(conf.SID, conf.AUTH_TOKEN, conf.TO_NUMBER, conf.FROM_NUMBER)
selling_price = 1000000.00#amount that is available with us (in rupees)
#getting details of bitcoin and ethereum cryptocurrencies in rupees,us dollars,euros
cryptocompare.get_price(['BTC','ETH'],['INR','USD','EUR'])
def price_check(b):
if(b=="b"):
p=cryptocompare.get_price('BTC',curr='INR')#to get the value of bitcoin in rupees
else:
p=cryptocompare.get_price('ETH',curr='INR')#to get value of ethereum in rupees
#converting price data type(p) into float datatype
for i in p.values():
pr=i
for t in pr.values():
return t
while True:
bitcoin_market_price = price_check("b")
ethereum_market_price=price_check("e")
print ('market price is: ',bitcoin_market_price)
print ('market price is: ',ethereum_market_price)
print ('Selling price is: ',selling_price)
try:
if bitcoin_market_price < selling_price:#indicates u can buy both currencies by both led's glow
bolt.digitalWrite("0","HIGH")#buzzer notifies
bolt.digitalWrite("1","HIGH")#LED'S GLOW
bolt.digitalWrite("2","HIGH")
print("Making request to Twilio to send a SMS")
response = sms.send_sms("bit coin and ethereum coin are available at prices "+str(bitcoin_market_price))
print("Response received from Twilio is: " + str(response))
print("Status of SMS at Twilio is :" + str(response.status))
elif ethereum_market_price < selling_price :#one led glow means u can buy ethereum but not bit coin
bolt.digitalWrite("0","HIGH")#buzzer
bolt.digitalWrite("1","HIGH")
bolt.digitalWrite("2","LOW")
print("Making request to Twilio to send a SMS")
response = sms.send_sms("ethereum is available at price "+str(ethereum_market_price))
print("Response received from Twilio is: " + str(response))
print("Status of SMS at Twilio is :" + str(response.status))
except Exception as e:
print ("Error occured: Below are the details")
print (e)
time.sleep(10)
bolt.digitalWrite("0","LOW")
bolt.digitalWrite("1","LOW")
bolt.digitalWrite("2","LOW")
conf
Pythonthis file contains details from twilio account so that we can send sms notification and details of our bolt device so that connect to bolt cloud api.
SID = 'your sid'
AUTH_TOKEN = 'your token fron twilio'
FROM_NUMBER = 'number from which message sent twilio account number'
TO_NUMBER = 'your number to which needed to be notified'
API_KEY = 'bolt cloud key'
DEVICE_ID = 'bolt device id'
Comments
Please log in or sign up to comment.