Hardware components | ||||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
| × | 1 | ||||
| × | 1 | ||||
| × | 1 | ||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
| × | 1 | ||||
![]() |
| × | 1 | |||
| × | 1 | ||||
Software apps and online services | ||||||
![]() |
| |||||
![]() |
| |||||
Hand tools and fabrication machines | ||||||
![]() |
|
This project allows the user to control the direction of the car through the coding of an Arduino and MIT app inventor. Building and coding this RC car is a great learning experience for anyone who would like to learn how to use an Arduino and learn the basics behind MIT app inventor. The project also allows the creator to control the car's movements on their phone. I hope you enjoy this project.
Schematic
Attaching the jumper cables:
Connect 5v on the arduino to the breadboard
Connect GND on the arduino to the breadboard
Connect enable1,2 to digital pin 11
Connect input 1 to digital pin 8
Connect output 1 to the negative of the hobby gearmotor
Connect GND(4) to ground on the breadboard
Connect output 2 to positive of the hobby gearmotor
Connect input 2 to digital pin 7
Connect Vcc 2 to 9v battery
Connect enable 3,4 to digital pin 3
Connect input 3 to digital pin 4
Connect output 3 to the positive of the second hobby gearmotor
Connect GND(13) to ground on the breadboard
Connect output 4 to the negative of the second hobby gearmotor
Connect Vcc 1 to 5v on the breadboard
Connect input 4 to digital pin 5
Connecting the Bluetooth module:
Connect Vcc to the power on the breadboard
Connect GND to the ground on the breadboard
Connect RXD to digital pin 1
Connect TXD to digital pin 0
Connecting the Ultrasonic Range Finder:
Connect GND to ground on the breadboard
Connect 5v to power on the breadboard
Connect echo to digital pin 10
Connect trig to digital pin 9
Connect 5v on the arduino to the breadboard
Connect GND on the arduino to the breadboard
Connect enable1,2 to digital pin 11
Connect input 1 to digital pin 8
Connect output 1 to the negative of the hobby gearmotor
Connect GND(4) to ground on the breadboard
Connect output 2 to positive of the hobby gearmotor
Connect input 2 to digital pin 7
Connect Vcc 2 to 9v battery
Connect enable 3,4 to digital pin 3
Connect input 3 to digital pin 4
Connect output 3 to the positive of the second hobby gearmotor
Connect GND(13) to ground on the breadboard
Connect output 4 to the negative of the second hobby gearmotor
Connect Vcc 1 to 5v on the breadboard
Connect input 4 to digital pin 5
Connecting the Bluetooth module:
Connect Vcc to the power on the breadboard
Connect GND to the ground on the breadboard
Connect RXD to digital pin 1
Connect TXD to digital pin 0
Connecting the Ultrasonic Range Finder:
Connect GND to ground on the breadboard
Connect 5v to power on the breadboard
Connect echo to digital pin 10
Connect trig to digital pin 9
_IGZlBcDLje.png)
Sketch
It is good draw a project for a physical visualization and to label each component for clarity.
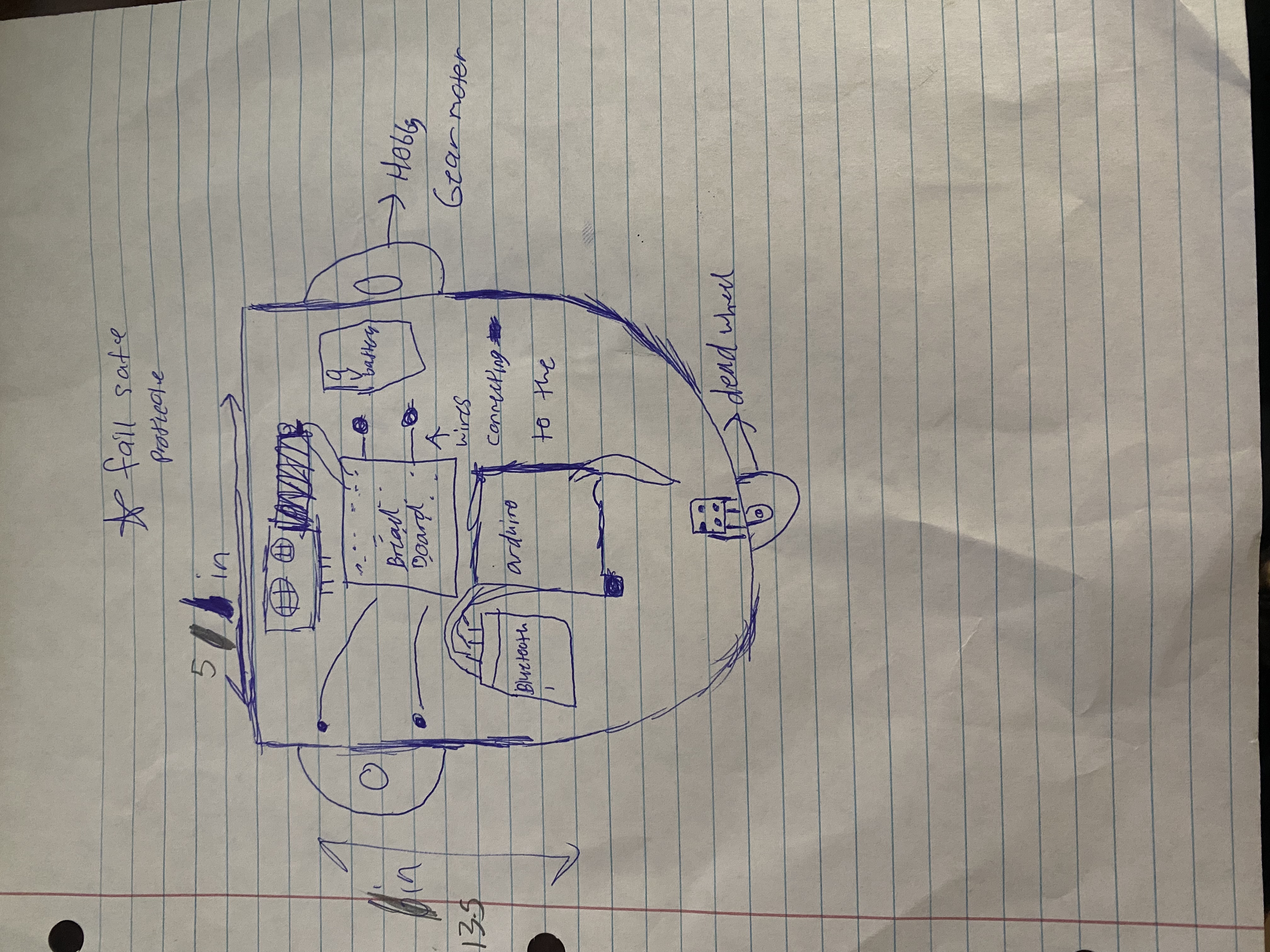
Prototype
Before building the final product of your project it is important to prototype the project. Through building this project on a smaller scale or a cheaper material you can adequately size and organize each part of the project.
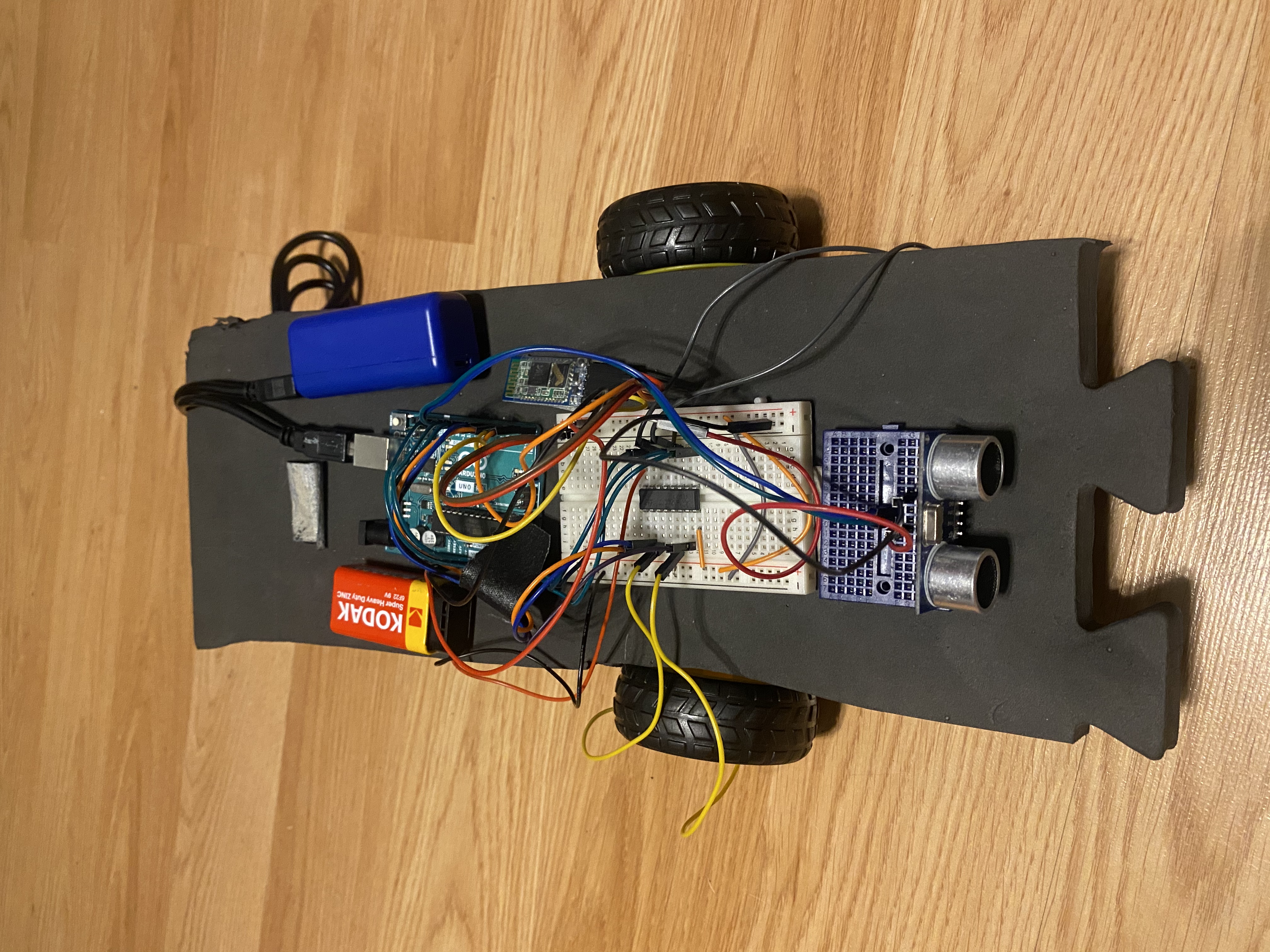
Style
Even though this is not an essential component of this project I chose to paint the wooden base that I decided to use. I did this to add my aspect to this project.
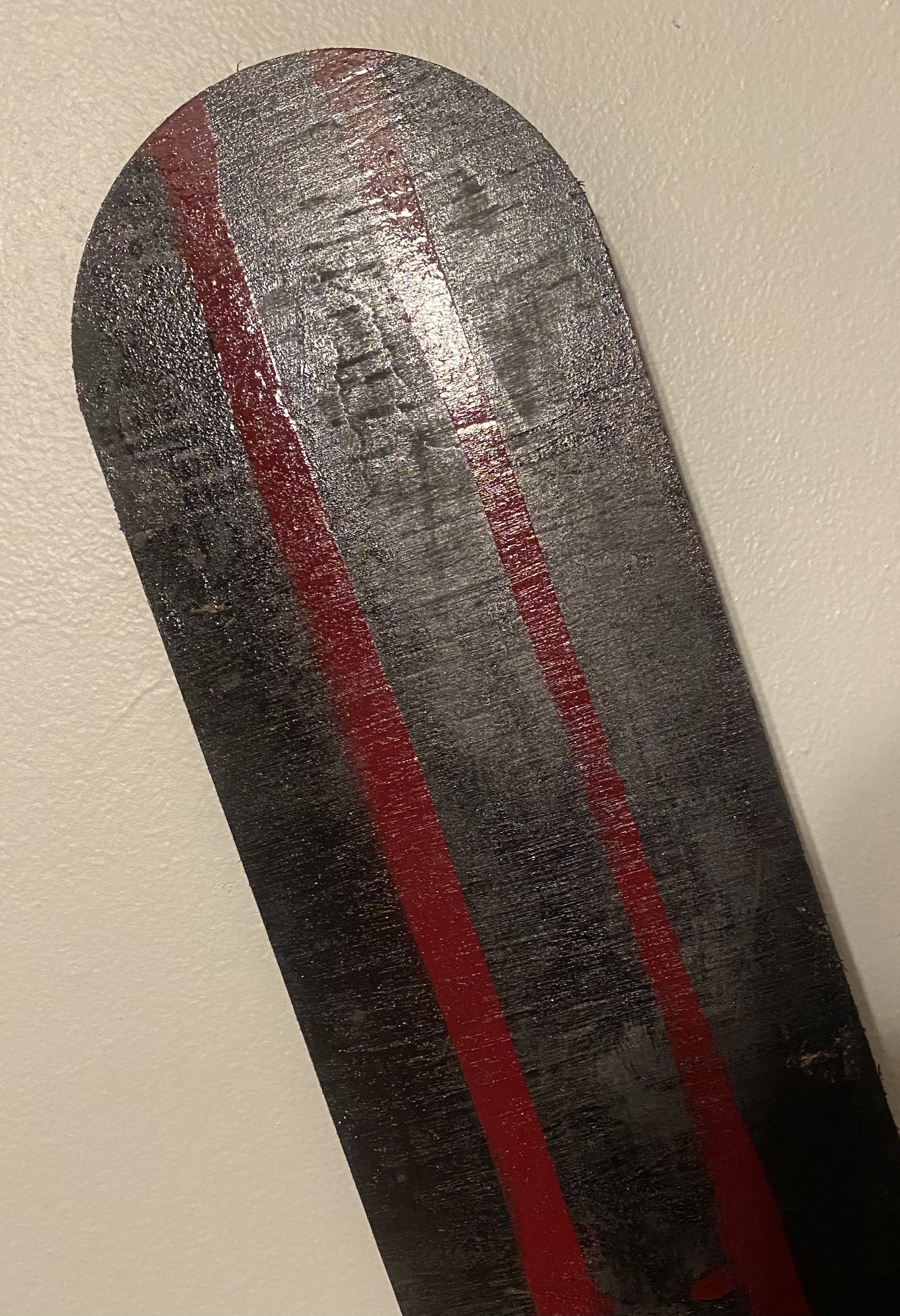
Remote Controlled car with emergency brake
ArduinoRemember to disconnect the Bluetooth module when uploading code to the Arduino board.
const int OrgEnable = 3;// yellow motor
const int TealIn =4; // yellow motor input 4
const int PurpIn = 5;// yellow motor input 3
const int Greenenb= 11; // grey motor
const int GreenA = 8; // grey motor input 1
const int GreenB =7;// grey motor input 2
char command;
int inches = 0;
int cm = 0;
#include <NewPing.h>
#define TRIGGER_PIN 9
#define ECHO_PIN 10
#define MAX_DISTANCE 200
NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE);
float duration, distance;
void setup()
{
Serial.begin(9600);
pinMode(OrgEnable, OUTPUT);
pinMode(TealIn, OUTPUT);
pinMode(PurpIn, OUTPUT);
pinMode(A5,INPUT);
digitalWrite(OrgEnable,LOW);
digitalWrite( TealIn, LOW);
digitalWrite( PurpIn, LOW);
pinMode(Greenenb, OUTPUT);
pinMode(GreenA, OUTPUT);
pinMode(GreenB, OUTPUT);
digitalWrite(Greenenb,LOW);
digitalWrite( GreenA, LOW);
digitalWrite( GreenB, LOW);
}
void loop()
{
// Send ping, get distance in cm
distance = sonar.ping_cm();
Serial.print(distance);
// Send results to Serial Monitor
Serial.print("Distance = ");
if (distance <= 12 )
{
digitalWrite(TealIn,LOW);
digitalWrite(PurpIn,LOW);
// delay(10);
digitalWrite(GreenA,LOW);
digitalWrite(GreenB,LOW);
Serial.print(distance);
Serial.println(" cm");
}
else
{
Serial.println("Out of range");
}
delay(50);
if(Serial.available()){
command = Serial.read();
Serial.println(command);
FowardM1();
FowardM2();
delay(20);
Right();
delay(20);
OffM2();
delay(20);
left();
delay(20);
ReverseM1();
ReverseM2();
delay(20);
OffM1();
}
}
void FowardM1(){ // motor 1 is M1
if (command==1){
digitalWrite(TealIn,HIGH);
digitalWrite(PurpIn,LOW);
// ramp up
for(int counter= 0;counter<=255;counter ++){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
}
}
void FowardM2(){ // M2 is motor 2
if (command==1){
digitalWrite(GreenA,HIGH);
digitalWrite(GreenB,LOW);
// ramp up
for(int counter= 0;counter<=255;counter ++){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
}
}
void Right(){
if (command==4){
digitalWrite(TealIn,HIGH);
digitalWrite(PurpIn,LOW);
// ramp up
for(int counter= 0;counter<=255;counter ++){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
delay(20);
digitalWrite(GreenA,LOW);
digitalWrite(GreenB,LOW);
}
}
void OffM2(){
if (command==0){
digitalWrite(GreenA,LOW);
digitalWrite(GreenB,LOW);
}
}
void left(){
if (command==3){
digitalWrite(GreenA,HIGH);
digitalWrite(GreenB,LOW);
// ramp up
for(int counter= 0;counter<=255;counter ++){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
delay(20);
digitalWrite(TealIn,LOW);
digitalWrite(PurpIn,LOW);
}
}
void ReverseM1 (){
if (command==2){
digitalWrite(TealIn,LOW);
digitalWrite(PurpIn,HIGH);
for(int counter= 255;counter<=0;counter --){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
}
}
void ReverseM2(){
if (command==2){
digitalWrite(GreenA,LOW);
digitalWrite(GreenB,HIGH);
for(int counter= 255;counter<=0;counter --){
analogWrite(OrgEnable,counter); // yellow motor on
analogWrite(Greenenb,counter);
}
}
}
void OffM1(){
if (command==0){
digitalWrite(TealIn,LOW);
digitalWrite(PurpIn,LOW);
}
}
Comments
Please log in or sign up to comment.