Hardware components | ||||||
![]() |
| × | 2 | |||
![]() |
| × | 1 | |||
| × | 1 | ||||
| × | 5 | ||||
Hand tools and fabrication machines | ||||||
![]() |
|
I received this mannequin as a gift. After a face-plant, some of his electronics and mechanical guts were missing. He has an on-board computer that drives his original sound functions. I wanted to restore his jaw and neck operation. It evolved from there as I learned more and more.
line level dc bias circuit
sound/speaker wires carry an alternating current from negative 5 volts to positive 5 volts. It's the negative voltage that would damage an arduino input. This circuit sends one speaker wire to ground and the other speaker wire goes in between two resistors on a steady 5 volt supply. The signal wire goes in between the resistors as well. The resistors I used were 10k Ohm. I tried 100k Ohm and immediately had signal issues.
The 10uF 16v capacitor acts as a bit of a filter for the sound signal by sitting on the negative speaker wire.
The 10uF 16v capacitor acts as a bit of a filter for the sound signal by sitting on the negative speaker wire.
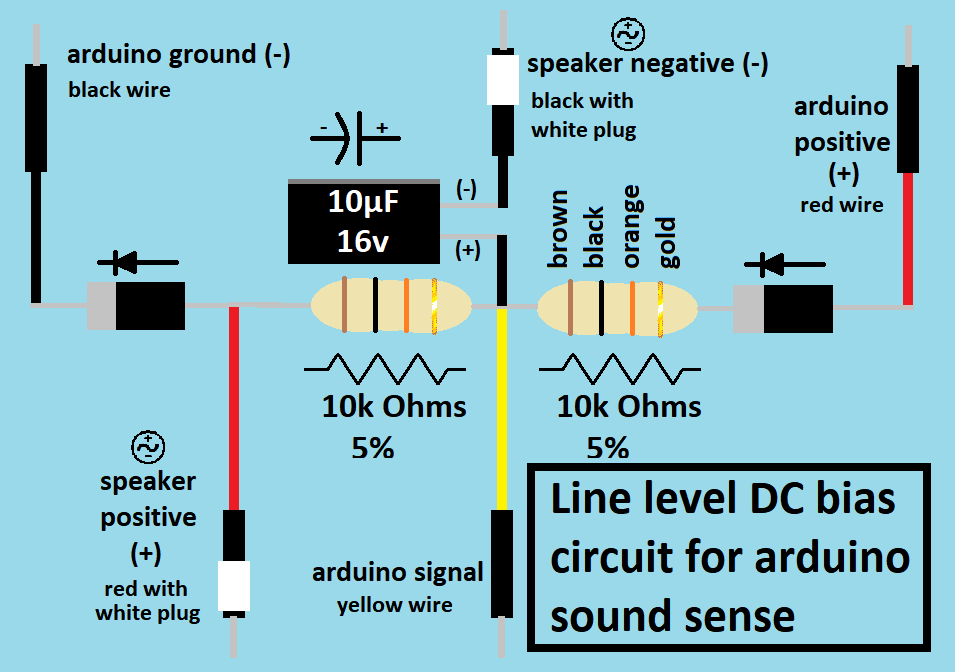
- 25102018 night mega code PAGES UPDATED!!!
- 24102018 Mega code
- 24102018 mouth code
- 22102018 graves uno head code late night
- 22102018 graves mega master
- 22102018 graves uno mouth
- 22102018 graves uno head code
- 19102018 they are playing well together
- Torture works!
- do you expect me to talk?
- button groups refined
- buttons again
- 13102018 - Bodmer to the rescue!
- 12102018 later in the day
- draft 12102018
- draft 2
- very rough draft
25102018 night mega code PAGES UPDATED!!!
C/C++Man, that was tough! All pages updated and coupled to button groups. button groups updated to accommodate backlight timer adjustment
/*
25102018
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
10k Ohm \ wire) /
resistor \ /
*--------/\/\/---------*---------/\/\/--------*
| \ 10k Ohm |
| \ resistor |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ -)|-
\ 100uF 16v
\ capacitor
\
\
speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//#include <Wire.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
char incomingMouthByte;
char outgoingMouthByte;
int mouthOnOffNew;
int mouthOnOffOld;
int mouthOnOffDifference;
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffNew;
int headOnOffOld;
int headOnOffDifference;
int headOnOffAdjusted; // value adjusted by 48 for ascii
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
Serial1.begin(9600);
Serial2.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 1;
outgoingHeadByte = 1; // for a bit more talkative serial data
headOnOffNew = 1; // stores head state from serial connection
headOnOffOld = 1; // stores head state from serial connection
incomingMouthByte = 0;
outgoingMouthByte = 0; // for a bit more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
////////////////////////////////
//// manually set variables ////
////////////////////////////////
// \/ \/ \/ \/ \/ \/
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
// /\ /\ /\ /\ /\ /\
////////////////////////////////
//// manually set variables ////
////////////////////////////////
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){ // most regular pages
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){ // on off buttons
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
mouthUnoTxRx();
}
}
if(buttonGroup == 2){ // head buttons
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
headUnoTxRx();
}
}
if(buttonGroup == 3){ // head buttons
tftButtonGroup3(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOffNew = 1; // on
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOffNew = 2; // off
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// mouthOnOffNew = 3; // off
// Serial.print("mouthOnOffNew set to ");
// Serial.println(mouthOnOffNew);
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOffNew = 1; // on
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
headOnOffNew = 2; // off
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup3----------------------------------
//--------------------------begin tftButtonGroup3----------------------------------
//--------------------------begin tftButtonGroup3----------------------------------
//--------------------------begin tftButtonGroup3----------------------------------
int tftButtonGroup3(){ // for tft backlight
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
if(75<p.x && p.x<140){
//Button 1
if(100<p.y && p.y<164){
backlightTimer = 120000;
}
//Button 2
if(164<p.y && p.y<240){
backlightTimer = 15000;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
backlightTimer = 5000;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup3
//--------------------------end tftButtonGroup3 code----------------------------------
//--------------------------end tftButtonGroup3 code----------------------------------
//--------------------------end tftButtonGroup3 code----------------------------------
//--------------------------end tftButtonGroup3 code----------------------------------
//--------------------------end tftButtonGroup3 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
if(mouthOnOffNew != mouthOnOffOld){
Serial.println("starting txrx");
if(mouthOnOffNew == 1){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('1');
mouthOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 1: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 2){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('2');
mouthOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 2: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 3){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('3');
mouthOnOffOld = 3;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 3: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 4){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('4');
mouthOnOffOld = 4;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 4: ");
Serial.println(mouthOnOffAdjusted);
}
else if(mouthOnOffAdjusted <= 1 && 4 >= mouthOnOffAdjusted){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted: ");
Serial.println(mouthOnOffAdjusted);
}
}
else if(mouthOnOffOld = mouthOnOffNew){
}
Serial.flush();
Serial1.flush();
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx----------------------------------
void headUnoTxRx(){
if(headOnOffNew != headOnOffOld){
Serial.println("starting txrx");
if(headOnOffNew == 1){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('1');
headOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin instead of delay
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 1: ");
Serial.println(headOnOffAdjusted);
}
if(headOnOffNew == 2){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('2');
headOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 2: ");
Serial.println(headOnOffAdjusted);
}
else if(headOnOffAdjusted != 1 && headOnOffAdjusted != 2){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted: ");
Serial.println(headOnOffAdjusted);
}
}
else if(headOnOffOld = headOnOffNew){
}
Serial.flush();
Serial2.flush();
}
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(70, 100, 60, 30, BLACK);
Tft.drawNumber((buttonGroup), 70, 100, 3, GREEN);
Tft.fillRectangle(140, 100, 60, 30, BLACK);
Tft.drawNumber((mouthOnOffNew), 140, 100, 3, GREEN);
Tft.fillRectangle(160, 100, 60, 30, BLACK);
Tft.drawNumber((headOnOffNew), 160, 100, 3, GREEN);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
screen 0000 A)Main Controls
screen 0001 a)motor controls
screen 0001 b) Touch Screen Timeout
screen 0011 -longer
screen 0011 -shorter
screen 0011 -reset
screen 0001 c)sensor monitors
screen 0021 -head reports
screen 0121 *report pir detection
screen 0121 *report pot position
screen 0021 -mouth reports
screen 0221 *graves sense
screen 0221 *echo sense
screen 0221 *report mouth servo position
// screen 0221 *adjust sensitivity?
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0002 b)sound sensors on/off
screen 0022 -echo sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -echo
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)head motor on/off
screen 0013 -on
screen 0013 -off
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
if(currentScreen != 31){
z = z + 300;
Serial.println("z + 300");
}
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
//================button group exceptions================
//================button group exceptions================
if(currentScreen == 111){ // HEAD MOTOR CONTOL
buttonGroup=1;
}
if(currentScreen == 12){ // MOUTH MOTOR CONTOL
buttonGroup=1;
}
if(currentScreen == 13){ // HEAD MOTOR CONTOL
buttonGroup=2;
}
if(currentScreen == 211){ // MOUTH MOTOR CONTOL
buttonGroup=2;
}
if(currentScreen == 31){ // BACKLIGHTIMER
buttonGroup=3;
}
//================button group exceptions================
//================button group exceptions================
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",18,50,4,GREEN);
Tft.drawString("Main Controls", 05,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, CYAN);
Tft.drawString("Head Control", 10, 260, 3, BLUE);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MAIN", 65, 5, 4, GREEN);
Tft.drawString("CONTROLS", 25, 50, 4, GREEN);
Tft.drawString("Motors Power", 15, 130, 3, RED);
Tft.drawString("Sensors", 55, 195, 3, YELLOW);
Tft.drawString("Light Timeout", 5, 260, 3, WHITE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MOTOR", 60, 5, 4, RED);
Tft.drawString("CONTROLS", 20, 50, 4, RED);
Tft.drawString("Head Motor", 30, 130, 3, BLUE);
Tft.drawString("Mouth Motor", 25, 195, 3, CYAN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0111 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 111){
// " "
Tft.drawString("HEAD MOTOR",0,5,4,BLUE);
Tft.drawString("CONTROL", 40, 50, 4, BLUE);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0111 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0211 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 211){
// " "
Tft.drawString("MOUTH", 60, 5, 4, CYAN);
Tft.drawString("MOTOR", 60, 50, 4, CYAN);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0211 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("BACKLIGHT",10,5,4,WHITE);
Tft.drawString("TIME OUT",20,50,4,WHITE);
Tft.drawString("2 minutes", 35,130, 3, GREEN);
Tft.drawString("15 seconds", 30, 195, 3, RED);
Tft.drawString("5 seconds", 35, 260, 3, WHITE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("INCOMING", 20, 5, 4, GREEN);
Tft.drawString("SENSORS", 30, 50, 4,YELLOW);
Tft.drawString("Head Sensors", 15, 130, 3, YELLOW);
Tft.drawString("Mouth Sensors", 05, 195, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("HEAD", 65, 5, 4, BLUE);
Tft.drawString("SENSORS", 30, 50, 4, YELLOW);
Tft.drawString("PIR position ", 10, 130, 3, YELLOW);
Tft.drawString("detected", 05, 195, 3, YELLOW);
Tft.drawChar(incomingHeadByte, 185, 195, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("MOUTH", 55, 5, 4, CYAN);
Tft.drawString("SENSORS", 30, 50, 4, YELLOW);
Tft.drawString("Echo Sense", 30, 130, 3, YELLOW);
Tft.drawString("Graves Sense", 10, 195, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("MOUTH", 50, 5, 4, CYAN);
Tft.drawString("CONTROL", 30, 50, 4, CYAN);
Tft.drawString("Motor on/off", 15, 130, 3, RED);
Tft.drawString("Sensor on/off", 05, 195, 3, YELLOW);
Tft.drawString("Monitor", 5, 260, 3, YELLOW);
Tft.drawChar(incomingMouthByte, 185, 260, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("MOUTH", 60, 5, 4, CYAN);
Tft.drawString("MOTOR", 60, 50, 4, CYAN);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("SENSOR", 40, 5, 4, YELLOW);
Tft.drawString("SELECT", 40, 50, 4,YELLOW);
Tft.drawString("Echo Sense", 30, 130, 3, YELLOW);
Tft.drawString("Graves Sense", 10, 195, 3, YELLOW);
Tft.drawString("Monitor Both", 10, 260, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("ECHO", 70, 5, 4, YELLOW);
Tft.drawString("SENSOR", 35, 50, 4, YELLOW);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("Monitor", 5, 260, 3, GREEN);
Tft.drawChar(incomingMouthByte, 185, 260, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("GRAVES",35,5,4,BLUE);
Tft.drawString("SENSOR",35,50,4,YELLOW);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("Monitor", 5, 260, 3, GREEN);
Tft.drawChar(incomingMouthByte, 185, 260, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("ALL", 90, 5, 4, BLUE);
Tft.drawString("SENSORS", 35, 50, 4, YELLOW);
Tft.drawString("Echo", 35, 130, 3, BLUE);
Tft.drawChar(incomingMouthByte, 185, 130, 3, YELLOW);
Tft.drawString("Graves", 30, 195, 3, GREEN);
Tft.drawChar(incomingMouthByte, 185, 130, 3, YELLOW);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("HEAD", 70, 5, 4, BLUE);
Tft.drawString("CONTROL", 30, 50, 4, RED);
Tft.drawString("Motor On/Off", 15, 130, 3, RED);
Tft.drawString("Manual Point", 15, 195, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("HEAD", 70, 5, 4, BLUE);
Tft.drawString("MOTOR", 60, 50, 4, RED);
Tft.drawString("On", 100, 130, 3, GREEN);
Tft.drawString("Off", 95, 195, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("HEAD", 70, 5, 4, BLUE);
Tft.drawString("POINTER", 35, 50, 4, RED);
Tft.drawString("Left", 85, 130, 3, RED);
Tft.drawString("Right", 75, 195, 3, RED);
Tft.drawString("Center", 65, 260, 3, RED);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
24102018 Mega code
C/C++Screens partially updated. New button groups need adjustment to couple correct screen assignments.
/*
24102018
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
10k Ohm \ wire) /
resistor \ /
*--------/\/\/---------*---------/\/\/--------*
| \ 10k Ohm |
| \ resistor |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ -)|-
\ 100uF 16v
\ capacitor
\
\
speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//#include <Wire.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
char incomingMouthByte;
char outgoingMouthByte;
int mouthOnOffNew;
int mouthOnOffOld;
int mouthOnOffDifference;
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffNew;
int headOnOffOld;
int headOnOffDifference;
int headOnOffAdjusted; // value adjusted by 48 for ascii
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
Serial1.begin(9600);
Serial2.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 1;
outgoingHeadByte = 1; // for a bit more talkative serial data
headOnOffNew = 1; // stores head state from serial connection
headOnOffOld = 1; // stores head state from serial connection
incomingMouthByte = 0;
outgoingMouthByte = 0; // for a bit more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){ // most regular pages
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){ // on off buttons
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
mouthUnoTxRx();
}
}
if(buttonGroup == 2){ // head buttons
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
headUnoTxRx();
}
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOffNew = 1; // on
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOffNew = 2; // off
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// mouthOnOffNew = 3; // off
// Serial.print("mouthOnOffNew set to ");
// Serial.println(mouthOnOffNew);
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOffNew = 1; // on
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
headOnOffNew = 2; // off
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
if(mouthOnOffNew != mouthOnOffOld){
Serial.println("starting txrx");
if(mouthOnOffNew == 1){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('1');
mouthOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 1: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 2){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('2');
mouthOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 2: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 3){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('3');
mouthOnOffOld = 3;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 3: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 4){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('4');
mouthOnOffOld = 4;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 4: ");
Serial.println(mouthOnOffAdjusted);
}
else if(mouthOnOffAdjusted <= 1 && 4 >= mouthOnOffAdjusted){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted: ");
Serial.println(mouthOnOffAdjusted);
}
}
else if(mouthOnOffOld = mouthOnOffNew){
}
Serial.flush();
Serial1.flush();
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx----------------------------------
void headUnoTxRx(){
if(headOnOffNew != headOnOffOld){
Serial.println("starting txrx");
if(headOnOffNew == 1){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('1');
headOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin instead of delay
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 1: ");
Serial.println(headOnOffAdjusted);
}
if(headOnOffNew == 2){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('2');
headOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 2: ");
Serial.println(headOnOffAdjusted);
}
else if(headOnOffAdjusted != 1 && headOnOffAdjusted != 2){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted: ");
Serial.println(headOnOffAdjusted);
}
}
else if(headOnOffOld = headOnOffNew){
}
Serial.flush();
Serial2.flush();
}
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(70, 100, 60, 30, BLACK);
Tft.drawNumber((buttonGroup), 70, 100, 3, GREEN);
Tft.fillRectangle(140, 100, 60, 30, BLACK);
Tft.drawNumber((mouthOnOffNew), 140, 100, 3, GREEN);
Tft.fillRectangle(160, 100, 60, 30, BLACK);
Tft.drawNumber((headOnOffNew), 160, 100, 3, GREEN);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Central Command
screen 0001 a)motor controls
screen 0001 b) Touch Screen Timeout
screen 0011 -longer
screen 0011 -shorter
screen 0011 -reset
screen 0001 c)sensor monitors
screen 0021 -head reports
screen 0121 *report pir detection
screen 0121 *report pot position
screen 0021 -mouth reports
screen 0221 *graves sense
screen 0221 *echo sense
screen 0221 *report mouth servo position
// screen 0221 *adjust sensitivity?
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0002 b)sound sensors on/off
screen 0022 -echo sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -echo
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)head motor on/off
screen 0013 -on
screen 0013 -off
screen 0003 b)manual point
screen 0023 -slider
---/\new screens/\---
---\/old screens\/---
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense echo sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -echo sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -echo
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
//================button group exceptions================
//================button group exceptions================
if(currentScreen == 122){
buttonGroup=1;
}
if(currentScreen == 222){
buttonGroup=1;
}
if(currentScreen == 23){
buttonGroup=2;
}
//================button group exceptions================
//================button group exceptions================
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Main Controls", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("SETTINGS",00,5,4,BLUE);
Tft.drawString("FOR THINGS",00,50,4,BLUE);
Tft.drawString("Motors Power", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Light Timeout", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("BACKLIGHT",00,5,4,BLUE);
Tft.drawString("TIME OUT",10,50,4,YELLOW);
Tft.drawString("Increase", 80,130, 3, BLUE);
Tft.drawString("Decrease", 05, 195, 3, GREEN);
Tft.drawString("Reset", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("INCOMING",00,5,4,BLUE);
Tft.drawString("SENSOR DATA",10,50,4,YELLOW);
Tft.drawString("Head Sensors", 80,130, 3, BLUE);
Tft.drawString("Mouth Sensors", 05, 195, 3, GREEN);
// Tft.drawString("Head Position", 10, 260, 3, GREEN);
// Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("HEAD",0,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("MOUTH",0,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("Echo Sense", 05, 195, 3, GREEN);
Tft.drawString("Servo Position", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("JAW TEST",0,5,4,BLUE);
Tft.drawString("OPERATION",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
// Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("SOUND SENSOR",0,5,4,BLUE);
Tft.drawString("SELECTION",10,50,4,YELLOW);
Tft.drawString("echo Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor Both", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("ECHO SENSOR",0,5,4,BLUE);
Tft.drawString("MONITOR",10,50,4,YELLOW);
Tft.drawString("", 120, 130, 3, GREEN);
Tft.drawString("", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("GRAVES SENSOR",0,5,4,BLUE);
Tft.drawString("MONITOR",10,50,4,YELLOW);
Tft.drawString("", 120, 130, 3, GREEN);
Tft.drawString("", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("ECHO & GRAVES",0,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("echo", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("HEAD MOTOR",0,5,4,BLUE);
Tft.drawString("CONTROL",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("GRAVES HEAD",0,5,3,BLUE);
Tft.drawString("POINTER",10,50,3,YELLOW);
Tft.drawString("Left", 120, 130, 3, GREEN);
Tft.drawString("Right", 115, 195, 3, RED);
Tft.drawString("Center", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
24102018 mouth code
C/C++New code for the mouth arduino is getting ready to accommodate a new button group and needs threshold adjustment for both graves and echo. new dc bias circuit built with proper resistors thus the need for code adjustment
/*
24102018
gravesunomouth
graves project
arduino Uno
Slave 1
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
10k Ohm \ wire) /
resistor \ /
*--------/\/\/---------*---------/\/\/--------*
| \ 10k Ohm |
| \ resistor |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ -)|-
\ 100uF 16v
\ capacitor
\
\
speaker -
(black from speaker)
*/
#include <Servo.h>
#include <SPI.h>
//---------------------begin declarations-----------------------
// for servo actions
Servo mouthServo;
// for gathering and mapping sound data
int soundPinGraves=A3; // incoming lead from graves dc bias circuit
int soundPinEcho=A4; // incoming lead from echo dc bias circuit
int soundDetectedEcho; // stores value from sound pin
int soundLevelEcho; // converted values that only include 512-1024
int soundMapEcho; // conversion from 512-1024 to 0-255
int servoMapEcho; // conversion from 0-255 to 100-70
int soundDetectedGraves; // stores value from sound pin
int soundLevelGraves; // converted values that only include 512-1024
int soundMapGraves; // conversion from 512-1024 to 0-255
int servoMapGraves; // conversion from 0-255 to 100-70
// communication
char incomingMouthByte; // for incoming serial data
char outgoingMouthByte; // for a bit more talkative serial data
int mouthOnOffNew; // stores mouth state from serial connection
int mouthOnOffOld; // stores mouth state from serial connection
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
// for blinking
const int ledPin = LED_BUILTIN;// the number of the LED pin
int ledState = LOW; // ledState used to set the LED
unsigned long previousMillis = 0; // will store last time LED was updated
//---------------------end declarations-----------------------
//*********************************************************
//*********************************************************
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
void setup(){
delay(1000);
Serial.begin(9600);
mouthServo.attach(6);
incomingMouthByte = 0; // for incoming serial data
outgoingMouthByte = 0; // for a little more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
pinMode(ledPin, OUTPUT);
pinMode(soundPinEcho, INPUT);
pinMode(soundPinGraves, INPUT);
}
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//**********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//**********************************************************
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
void loop(){
serialDoStuff();
if(mouthOnOffNew == mouthOnOffOld){
if(mouthOnOffNew == 1){
blinkFast();
mouthDoStuffGraves();
mouthDoStuffEcho();
}
if(mouthOnOffNew == 2){
mouthDoStuffEcho();
blinkSlow();
}
if(mouthOnOffNew == 3){
blinkSlow();
mouthDoStuffGraves();
}
if(mouthOnOffNew == 4){
}
if(mouthOnOffNew == 5){
}
if(mouthOnOffNew == 6){
}
if(mouthOnOffNew == 7){
}
if(mouthOnOffNew == 8){
}
}
else if(mouthOnOffNew != mouthOnOffOld){
mouthOnOffNew = mouthOnOffOld;
}
}
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//*********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*********************************************************
//---------------------begin serialDoStuff -----------------
void serialDoStuff(){
if(Serial.available() > 0){
incomingMouthByte = Serial.read();
mouthOnOffAdjusted = incomingMouthByte - '0';
if(mouthOnOffAdjusted == 1){
Serial.flush();
outgoingMouthByte = 1;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 1;
}
else if(mouthOnOffAdjusted == 2){
Serial.flush();
outgoingMouthByte = 2;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 2;
}
else if(mouthOnOffAdjusted == 3){
Serial.flush();
outgoingMouthByte = 3;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 3;
}
else if(mouthOnOffAdjusted == 4){
Serial.flush();
outgoingMouthByte = 4;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 4;
}
else if(mouthOnOffAdjusted == 5){
Serial.flush();
outgoingMouthByte = 5;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 5;
}
else if(mouthOnOffAdjusted == 6){
Serial.flush();
outgoingMouthByte = 6;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 6;
}
else if(mouthOnOffAdjusted == 7){
Serial.flush();
outgoingMouthByte = 7;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 7;
}
else if(mouthOnOffAdjusted == 8){
Serial.flush();
outgoingMouthByte = 8;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 8;
}
}
Serial.println(analogRead(soundPinGraves));
Serial.print(" ");
Serial.println(analogRead(soundPinEcho));
// Serial.print("raw LevelGraves ");
// Serial.print(analogRead(soundPinGraves));
// Serial.print("soundLevelGraves ");
// Serial.println(soundLevelGraves);
// Serial.print(" servoMapGraves ");
// Serial.println(servoMapGraves);
// Serial.print("soundDetectedGraves ");
// Serial.println(soundDetectedGraves);
}
//---------------------end serialDoStuff-----------------
//*********************************************************
//*********************************************************
//---------------------begin mouthDoStuffGraves -------------------
void mouthDoStuffGraves(){
int soundDetectedGraves; // stores value from sound pin
int soundLevelGraves; // converted values that only include 512-1024
int servoMapGraves; // conversion from 0-255 to 100-70
soundDetectedGraves = analogRead(soundPinGraves); // capture audio signal from dc bias circuit
if(soundDetectedGraves<=512){ // runs when negative amplitude detected
soundLevelGraves = (512-soundDetectedGraves+512); // takes numbers below 512 and tacks em on top of 512 to create positive amplitudes
}
else{
soundLevelGraves = soundDetectedGraves; // stores positive amplitudes
}
servoMapGraves = map(soundLevelGraves, 570, 610, 100, 50); // sound map 0-255 is mapped to truncated and inverted servo sweep 100-70
if(soundLevelGraves < 700 ){
if(servoMapGraves < 100){
mouthServo.write(servoMapGraves);
}
}
else{
mouthServo.write(95);
}
// return soundLevelGraves;
}
//// ++++++++++++++++++end mouthDoStuffGraves +++++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin mouthDoStuffEcho -------------------
void mouthDoStuffEcho(){
int soundDetectedEcho; // stores value from sound pin
int soundLevelEcho; // converted values that only include 512-1024
int servoMapEcho; // conversion from 0-255 to 100-70
soundDetectedEcho = analogRead(soundPinEcho); // capture audio signal from dc bias circuit
if(soundDetectedEcho<=512){ // runs when negative amplitude detected
soundLevelEcho = (512-soundDetectedEcho+512); // takes numbers below 512 and tacks em on top of 512 to create positive amplitudes
}
else{
soundLevelEcho = soundDetectedEcho; // stores positive amplitudes
}
servoMapEcho = map(soundLevelEcho, 570, 610, 100, 50); // sound map 0-255 is mapped to truncated and inverted servo sweep 100-70
if(soundLevelEcho < 700 ){
if(servoMapEcho < 100){
mouthServo.write(servoMapEcho);
}
}
else{
mouthServo.write(95);
}
// return soundLevelEcho;
}
//// ++++++++++++++++++end mouthDoStuffEcho +++++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin blink slow-------------------
void blinkSlow(){
unsigned long currentMillis = millis();
const long interval1 = 500;
if(currentMillis - previousMillis >= interval1) {
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink slow-------------------
//---------------------begin blink fast -------------------
void blinkFast(){
unsigned long currentMillis = millis();
const long interval2 = 75;
if(currentMillis - previousMillis >= interval2){
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink fast -------------------
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
22102018 graves uno head code late night
C/C++head is tracking better. head on off function control from tft is successful! added ramped speeds to motor control. mostly debugged. needs a trim. could talk a bit more and use its outgoing skills.
/*
22102018
gravesunohead
graves project
arduino Uno
Slave 2
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
Graves' head originally had a motor to look left and right. The routine failed; so, the built in
routine was scrapped in favor of a L298n motor controller coupled through the arduino to a pir
array added to the serving tray. The result is a passive tracking abilty to
face the skull towards the direction of the triggered sensor. CREEPY.
the pir sensors are hc-sr501 type passive infrared recievers with their bug-eye lenses removed
I bypassed R13 and R33 on all 5 sensors to decrease the hadwired delay.
the sensors need the addition of a pull-down reisistor circuit. The sensors send voltage to the pin
on the arduino when something is detected. When nothing is detected and the pins are left to float,
the system glitches with multiple false triggers. Thus, a pull down resistor is needed for every sensor
to ensure the state read is a 0.
signal in from pir signal continues to arduino digital pin
--------------------*-----------------------------------------
\
tap into signal wire \
\ 10k Ohm
\ resistor to ground (all bundled together to ground)
*-------/\/\/------------------------
*/
// L298n motor controller code
// connect motor controller pins to Arduino digital pins
// motor one
int enA = 10;
int in1 = 8;
int in2 = 9;
// L298n motor controller code
// pot switches
int potControlPin = A1; // analog pin used to connect the controller potentiometer
//int valControl; // variable to read the value from the analog pin for control input
int potControl = 0; // variable to store the servo position from the controller pot switch
int potPositionPin = A0; // analog pin used to connect the position monitoring potentiometer
int valPosition; // variable to read the value from the analog pin connected to position monitor
int potPosition = 0; // variable to store the servo position of the monitoring pot switch
// pot switches
int detectedPosition; // variable to store info
boolean pirStatus;
int servangle = 0; // servo angle variable
int pirNo[] = {3,4,5,6,7}; // pir pin numbers
int pirPrevLow[] = {1,1,1,1,1}; // previously low flag set to true
int pirPrevUsed[] = {0,0,0,0,0}; // has pir been on used before going low
unsigned long pirPrevMillis[] = {0,0,0,0,0}; // has pir been on used before going low
unsigned long pirCurMillis[] = {0,0,0,0,0}; // has pir been on used before going low
int pirPos[] = {10,60,100,140,170}; // positions for servo (0-180)
int curPosPir = 0;
int pirPin = 3;
int senseState3;
int senseState4;
int senseState5;
int senseState6;
int senseState7;
unsigned long currentMillis;
unsigned long time;
long ignoreTime;
// for blinking
const int ledPin = LED_BUILTIN;// the number of the LED pin
int ledState = LOW; // ledState used to set the LED
unsigned long previousMillis = 0; // will store last time LED was updated
long interval = 1; // interval at which to move servos (milliseconds)
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffAdjusted;
int headOnOffOld;
int headOnOffNew;
void setup() {
delay(500);
Serial.begin(9600);
incomingHeadByte = 0; // for incoming serial data
outgoingHeadByte = 0; // for a bit more talkative serial data
headOnOffAdjusted = 1;
headOnOffOld = 1; // stores head state from serial connection
headOnOffNew = 1; // stores head state from serial connection
time = millis();
// motor control setup code
// set all the motor control pins to outputs
pinMode(enA, OUTPUT);
pinMode(in1, OUTPUT);
pinMode(in2, OUTPUT);
// motor control setup code
pinMode(LED_BUILTIN, OUTPUT);
pinMode(pirNo[3], INPUT);
pinMode(pirNo[4], INPUT);
pinMode(pirNo[5], INPUT);
pinMode(pirNo[6], INPUT);
pinMode(pirNo[7], INPUT);
// blink led to denote ready
blinkSlow();
}
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
void loop(){
serialDoStuff();
if(headOnOffNew == headOnOffOld){
if(headOnOffNew == 1){
sensePositionFunction();
headTurn();
pirReport();
}
if(headOnOffNew == 2){
previousMillis = currentMillis;
// do Thing C
// turn motor off
analogWrite(enA, 0);
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
outgoingHeadByte = '-';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if(headOnOffNew != headOnOffOld){
headOnOffNew = headOnOffOld;
}
}
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//*********************************************************
//*********************************************************
//---------------------begin serialDoStuff -----------------
void serialDoStuff(){
if(Serial.available() > 0){
incomingHeadByte = Serial.read();
headOnOffAdjusted = incomingHeadByte - '0';
if(headOnOffAdjusted == 1){
Serial.flush();
// outgoingHeadByte = 1;
// Serial.write(outgoingHeadByte);
headOnOffOld = 1;
}
else if(headOnOffAdjusted == 2){
Serial.flush();
// outgoingHeadByte = 2;
// Serial.write(outgoingHeadByte);
headOnOffOld = 2;
}
}
}
//---------------------end serialDoStuff-----------------
//*********************************************************
//*********************************************************
//*********************************************************
//*********************************************************
// ++++++++++++++++++begin PIR function +++++++++++++++++++++++++++++++
void sensePositionFunction(){
ignoreTime = 4000;
for(int j=0;j<5;j++){ // do this for each PIR in the array
pirPin=pirNo[j];
pirCurMillis[j] = millis();
pirStatus = digitalRead(pirPin);
if(pirStatus == HIGH){ // while cycling through the array if pirNo[j] is HIGH, do this
if(pirPrevLow[j]){ // and If pirNo[j] was previously low, do this
if(curPosPir != pirPin && pirPrevUsed[j] == 0){ // if pirNo[j] is HIGH and different than curPosPir, move to new position
curPosPir = pirPin; // keep current PIR
pirPrevUsed[j] = 1;
if(ignoreTime > pirCurMillis[j] - pirPrevMillis[j]){ // example 4 sec > 30 sec - 28 sec
Serial.print(" pin skipped for being too early ");
Serial.println(curPosPir);
}
else if(ignoreTime <= pirCurMillis[j] - pirPrevMillis[j]){ // example 4 sec < 38 sec - 32 sec
detectedPosition = pirPin;
pirPrevMillis[j] = millis();
}
// return detectedPosition;
}
pirPrevLow[j] = 0; // pir is now recorded as not low
}
}
else{
pirPrevLow[j] = 1; // pir is now recorded as low
pirPrevUsed[j] = 0;
}
} // end j number of pirs loop
Serial.print(" detectedPosition ");
Serial.println(detectedPosition);
}
//+++++++++++++++++++++++end PIR function +++++++++++++++++++++++++++++++
void pirReport(){
senseState3 = digitalRead(3);
// Serial.println(millis()/1000);
Serial.print(" 3");
Serial.print(senseState3);
delay(5);
senseState4 = digitalRead(4);
// Serial.println(millis()/1000);
Serial.print(" 4");
Serial.print(senseState4);
senseState5 = digitalRead(5);
// Serial.println(millis()/1000);
Serial.print(" 5");
Serial.print(senseState5);
senseState6 = digitalRead(6);
// Serial.println(millis()/1000);
Serial.print(" 6");
Serial.print(senseState6);
senseState7 = digitalRead(7);
// Serial.println(millis()/1000);
Serial.print(" 7");
Serial.print(senseState7);
// delay(5);
//clear screen
Serial.println("");
// Serial.println("");
// Serial.println("");
// Serial.println("");
// Serial.println("");
// Serial.println("");
// Serial.println("");
//
// delay(50);
}
//*********************************************************
//*********************************************************
//---------------------begin headTurn -------------------
void headTurn() {
currentMillis = millis();
long motorStep = 50; // time for motor to run before switching off
// begin potservo section for potentiometer control
// int valControl = analogRead(A0); // reads the value of the potentiometer (value between 0 and 1023)
// valControl = map(valControl, 0, 1023, 0, 10); // first set refers to pot min/max second is servo map
int valControl = detectedPosition; // reads the value of the potentiometer (value between 0 and 1023)
valControl = map(valControl, 7, 3, 1, 5); // first number set refers to pir array pins, second is servo map
int valPosition = analogRead(A0); // reads value of position pot
valPosition = map(valPosition, 350, 650, 5, 1); // first min second max read from position potentiometer
// Serial.print("control ");
// Serial.println(valControl);
Serial.print(" valControl ");
Serial.println(valControl);
Serial.print(" reported position ");
Serial.println(valPosition);
Serial.print(" position ");
Serial.println(analogRead(A1));
// Serial.print(" raw pot pos ");
// Serial.println(analogRead(A0));
if (valPosition > valControl + 3){ // new position is far
if(currentMillis - previousMillis > interval){
previousMillis = currentMillis;
// set speed to __ out of possible range 80~255
analogWrite(enA, 140);
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
// delay(200);
// digitalWrite(in1, LOW);
outgoingHeadByte = '<<<';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if(valPosition < valControl - 3){ // new position is far
if(currentMillis - previousMillis > interval) {
previousMillis = currentMillis;
// do Thing B
// turn on motor A backward
// set speed to __ out of possible range 80~255
analogWrite(enA, 140);
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
// delay(200);
// digitalWrite(in2, LOW);
outgoingHeadByte = '>>>';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if (valPosition == valControl + 2){ // new position is getting close
if(currentMillis - previousMillis > interval){
previousMillis = currentMillis;
// set speed to __ out of possible range 80~255
analogWrite(enA, 100);
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
// delay(100);
// digitalWrite(in1, LOW);
outgoingHeadByte = '<<';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if(valPosition == valControl - 2){ // new position is getting close
if(currentMillis - previousMillis > interval) {
previousMillis = currentMillis;
// do Thing B
// turn on motor A backward
// set speed to __ out of possible range 80~255
analogWrite(enA, 100);
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
// delay(100);
// digitalWrite(in2, LOW);
outgoingHeadByte = '>>';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if (valPosition == valControl + 1){ // new position is next over
if(currentMillis - previousMillis > interval){
previousMillis = currentMillis;
// set speed to __ out of possible range 80~255
analogWrite(enA, 85);
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
// delay(100);
// digitalWrite(in1, LOW);
outgoingHeadByte = '<';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else if(valPosition == valControl - 1){ // new position is next over
if(currentMillis - previousMillis > interval) {
previousMillis = currentMillis;
// do Thing B
// turn on motor A backward
// set speed to __ out of possible range 80~255
analogWrite(enA, 85);
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
// delay(100);
// digitalWrite(in2, LOW);
outgoingHeadByte = '>';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
else
{
previousMillis = currentMillis;
// do Thing C
// turn motor off
analogWrite(enA, 0);
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
outgoingHeadByte = '-';
Serial.write(outgoingHeadByte);
Serial.println("");
}
}
//---------------------end headTurn--------------------
//*********************************************************
//*********************************************************
//---------------------begin blink slow-------------------
void blinkSlow(){
unsigned long currentMillis = millis();
const long interval1 = 500;
if(currentMillis - previousMillis >= interval1) {
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink slow-------------------
//---------------------begin blink fast -------------------
void blinkFast(){
unsigned long currentMillis = millis();
const long interval2 = 75;
if(currentMillis - previousMillis >= interval2){
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink fast -------------------
22102018 graves mega master
C/C++code for arduino 2560 contols tft along with sending on off commands to head uno and mouth uno
/*
last edit monday oct 22 2018
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
\ wire) /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//#include <Wire.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
char incomingMouthByte;
char outgoingMouthByte;
int mouthOnOffNew;
int mouthOnOffOld;
int mouthOnOffDifference;
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffNew;
int headOnOffOld;
int headOnOffDifference;
int headOnOffAdjusted; // value adjusted by 48 for ascii
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
Serial1.begin(9600);
Serial2.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 1;
outgoingHeadByte = 1; // for a bit more talkative serial data
headOnOffNew = 1; // stores head state from serial connection
headOnOffOld = 1; // stores head state from serial connection
incomingMouthByte = 0;
outgoingMouthByte = 0; // for a bit more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){ // most regular pages
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){ // on off buttons
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
mouthUnoTxRx();
}
}
if(buttonGroup == 2){ // head buttons
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
headUnoTxRx();
}
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOffNew = 1; // on
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOffNew = 2; // off
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOffNew = 1; // on
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
headOnOffNew = 2; // off
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
if(currentScreen == 122){
buttonGroup=1;
}
if(currentScreen == 222){
buttonGroup=1;
}
if(currentScreen == 23){
buttonGroup=2;
}
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
if(mouthOnOffNew != mouthOnOffOld){
Serial.println("starting txrx");
if(mouthOnOffNew == 1){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('1');
mouthOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 1: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 2){
Serial.println("Starting tx");
Serial1.flush();
Serial1.write('2');
mouthOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 2: ");
Serial.println(mouthOnOffAdjusted);
}
else if(mouthOnOffAdjusted != 1 && mouthOnOffAdjusted != 2){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted: ");
Serial.println(mouthOnOffAdjusted);
}
}
else if(mouthOnOffOld = mouthOnOffNew){
}
Serial.flush();
Serial1.flush();
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx----------------------------------
void headUnoTxRx(){
if(headOnOffNew != headOnOffOld){
Serial.println("starting txrx");
if(headOnOffNew == 1){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('1');
headOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin instead of delay
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 1: ");
Serial.println(headOnOffAdjusted);
}
if(headOnOffNew == 2){
Serial.println("Starting tx");
Serial2.flush();
Serial2.write('2');
headOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted should be 2: ");
Serial.println(headOnOffAdjusted);
}
else if(headOnOffAdjusted != 1 && headOnOffAdjusted != 2){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(10); // tweak delay to accomodate uno
incomingHeadByte = Serial2.read();
Serial2.flush();
headOnOffAdjusted = incomingHeadByte;
Serial.print("headOnOffAdjusted: ");
Serial.println(headOnOffAdjusted);
}
}
else if(headOnOffOld = headOnOffNew){
}
Serial.flush();
Serial2.flush();
}
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(70, 100, 60, 30, BLACK);
Tft.drawNumber((buttonGroup), 70, 100, 3, GREEN);
Tft.fillRectangle(140, 100, 60, 30, BLACK);
Tft.drawNumber((mouthOnOffNew), 140, 100, 3, GREEN);
Tft.fillRectangle(160, 100, 60, 30, BLACK);
Tft.drawNumber((headOnOffNew), 160, 100, 3, GREEN);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Graves Head",0,5,3,BLUE);
Tft.drawString("Operations",10,50,3,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
// Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
/*
last edit monday oct 22 2018
gravesunomouth
graves project
arduino Uno
Slave 1
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
\ wire) /
\ /
*--------[=]-----------*---------[=]----------*
| 100k resistor \ 100k resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
#include <Servo.h>
#include <SPI.h>
//---------------------begin declarations-----------------------
// for servo actions
Servo mouthServo;
// for gathering and mapping sound data
int soundPinGraves=A3; // incoming lead from graves dc bias circuit
int soundPinEcho=A4; // incoming lead from echo dc bias circuit
int soundDetectedEcho; // stores value from sound pin
int soundLevelEcho; // converted values that only include 512-1024
int soundMapEcho; // conversion from 512-1024 to 0-255
int servoMapEcho; // conversion from 0-255 to 100-70
int soundDetectedGraves; // stores value from sound pin
int soundLevelGraves; // converted values that only include 512-1024
int soundMapGraves; // conversion from 512-1024 to 0-255
int servoMapGraves; // conversion from 0-255 to 100-70
// communication
char incomingMouthByte; // for incoming serial data
char outgoingMouthByte; // for a bit more talkative serial data
int mouthOnOffNew; // stores mouth state from serial connection
int mouthOnOffOld; // stores mouth state from serial connection
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
// for blinking
const int ledPin = LED_BUILTIN;// the number of the LED pin
int ledState = LOW; // ledState used to set the LED
unsigned long previousMillis = 0; // will store last time LED was updated
//---------------------end declarations-----------------------
//*********************************************************
//*********************************************************
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
void setup(){
delay(5000);
Serial.begin(9600);
mouthServo.attach(6);
incomingMouthByte = 0; // for incoming serial data
outgoingMouthByte = 0; // for a little more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
pinMode(ledPin, OUTPUT);
pinMode(soundPinEcho, INPUT);
pinMode(soundPinGraves, INPUT);
}
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//**********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//**********************************************************
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
void loop(){
serialDoStuff();
if(mouthOnOffNew == mouthOnOffOld){
if(mouthOnOffNew == 1){
blinkFast();
mouthDoStuffGraves();
mouthDoStuffEcho();
}
if(mouthOnOffNew == 2){
blinkSlow();
}
}
else if(mouthOnOffNew != mouthOnOffOld){
mouthOnOffNew = mouthOnOffOld;
}
}
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//*********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*********************************************************
//---------------------begin serialDoStuff -----------------
void serialDoStuff(){
if(Serial.available() > 0){
incomingMouthByte = Serial.read();
mouthOnOffAdjusted = incomingMouthByte - '0';
if(mouthOnOffAdjusted == 1){
Serial.flush();
outgoingMouthByte = 1;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 1;
}
else if(mouthOnOffAdjusted == 2){
Serial.flush();
outgoingMouthByte = 2;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 2;
}
/*
else if(mouthOnOffAdjusted == 3){
Serial.flush();
outgoingMouthByte = 3;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 3;
}
else if(mouthOnOffAdjusted == 4){
Serial.flush();
outgoingMouthByte = 4;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 4;
}
*/
}
}
//---------------------end serialDoStuff-----------------
//*********************************************************
//*********************************************************
//---------------------begin mouthDoStuffGraves -------------------
void mouthDoStuffGraves(){
int soundDetectedGraves; // stores value from sound pin
int soundLevelGraves; // converted values that only include 512-1024
int servoMapGraves; // conversion from 0-255 to 100-70
soundDetectedGraves = analogRead(soundPinGraves); // capture audio signal from dc bias circuit
if(soundDetectedGraves<=512){ // runs when negative amplitude detected
soundLevelGraves = (512-soundDetectedGraves+512); // takes numbers below 512 and tacks em on top of 512 to create positive amplitudes
}
else{
soundLevelGraves = soundDetectedGraves; // stores positive amplitudes
}
servoMapGraves = map(soundLevelGraves, 570, 610, 100, 50); // sound map 0-255 is mapped to truncated and inverted servo sweep 100-70
if(soundLevelGraves < 700 ){
if(servoMapGraves < 100){
mouthServo.write(servoMapGraves);
Serial.print("soundLevelGraves ");
Serial.println(soundLevelGraves);
Serial.print(" servoMapGraves ");
Serial.println(servoMapGraves);
Serial.print(" soundDetectedGraves ");
Serial.println(soundDetectedGraves);
}
}
else{
mouthServo.write(95);
Serial.print("soundLevelGraves ");
Serial.println(soundLevelGraves);
Serial.print(" servoMapGraves ");
Serial.println(servoMapGraves);
Serial.print(" soundDetectedGraves ");
Serial.println(soundDetectedGraves);
}
// return soundLevelGraves;
}
//// ++++++++++++++++++end mouthDoStuffGraves +++++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin mouthDoStuffEcho -------------------
void mouthDoStuffEcho(){
int soundDetectedEcho; // stores value from sound pin
int soundLevelEcho; // converted values that only include 512-1024
int servoMapEcho; // conversion from 0-255 to 100-70
soundDetectedEcho = analogRead(soundPinEcho); // capture audio signal from dc bias circuit
if(soundDetectedEcho<=512){ // runs when negative amplitude detected
soundLevelEcho = (512-soundDetectedEcho+512); // takes numbers below 512 and tacks em on top of 512 to create positive amplitudes
}
else{
soundLevelEcho = soundDetectedEcho; // stores positive amplitudes
}
servoMapEcho = map(soundLevelEcho, 570, 610, 100, 50); // sound map 0-255 is mapped to truncated and inverted servo sweep 100-70
if(soundLevelEcho < 700 ){
if(servoMapEcho < 100){
mouthServo.write(servoMapEcho);
Serial.print("soundLevelEcho ");
Serial.println(soundLevelEcho);
Serial.print(" servoMapEcho ");
Serial.println(servoMapEcho);
Serial.print(" soundDetectedEcho ");
Serial.println(soundDetectedEcho);
}
}
else{
mouthServo.write(95);
Serial.print("soundLevelEcho ");
Serial.println(soundLevelEcho);
Serial.print(" servoMapEcho ");
Serial.println(servoMapEcho);
Serial.print(" soundDetectedEcho ");
Serial.println(soundDetectedEcho);
}
// return soundLevelEcho;
}
//// ++++++++++++++++++end mouthDoStuffEcho +++++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin blink slow-------------------
void blinkSlow(){
unsigned long currentMillis = millis();
const long interval1 = 500;
if(currentMillis - previousMillis >= interval1) {
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink slow-------------------
//---------------------begin blink fast -------------------
void blinkFast(){
unsigned long currentMillis = millis();
const long interval2 = 75;
if(currentMillis - previousMillis >= interval2){
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink fast -------------------
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
/*
last edit monday oct 22 2018
gravesunohead
graves project
arduino Uno
Slave 1
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
*/
// L298n motor controller code
// connect motor controller pins to Arduino digital pins
// motor one
int enA = 10;
int in1 = 8;
int in2 = 9;
// L298n motor controller code
// pot switches
//int potControlPin = A1; // analog pin used to connect the controller potentiometer
//int valControl; // variable to read the value from the analog pin for control input
//int potControl = 0; // variable to store the servo position from the controller pot switch
int potPositionPin = A0; // analog pin used to connect the position monitoring potentiometer
int valPosition; // variable to read the value from the analog pin connected to position monitor
int potPosition = 0; // variable to store the servo position of the monitoring pot switch
// pot switches
int detectedPosition; // variable to store info
boolean pirStatus;
int servangle = 0; // servo angle variable
int pirNo[] = {3,4,5,6,7}; // pir pin numbers
int pirPrevLow[] = {1,1,1,1,1}; // previously low flag set to true
int pirPrevUsed[] = {0,0,0,0,0}; // has pir been on used before going low
int pirPos[] = {10,60,100,140,170}; // positions for servo (0-180)
int curPosPir = 0;
int pirPin = 3;
unsigned long time;
// for blinking
const int ledPin = LED_BUILTIN;// the number of the LED pin
int ledState = LOW; // ledState used to set the LED
unsigned long previousMillis = 0; // will store last time LED was updated
long interval = 1; // interval at which to move servos (milliseconds)
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffAdjusted;
int headOnOffOld;
int headOnOffNew;
void setup() {
delay(5000);
Serial.begin(9600);
incomingHeadByte = 0; // for incoming serial data
outgoingHeadByte = 0; // for a bit more talkative serial data
headOnOffAdjusted = 1;
headOnOffOld = 1; // stores head state from serial connection
headOnOffNew = 1; // stores head state from serial connection
time = millis();
// motor control setup code
// set all the motor control pins to outputs
pinMode(enA, OUTPUT);
pinMode(in1, OUTPUT);
pinMode(in2, OUTPUT);
// motor control setup code
pinMode(LED_BUILTIN, OUTPUT);
// blink led to denote ready
blinkSlow();
}
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
void loop(){
serialDoStuff();
if(headOnOffNew == headOnOffOld){
if(headOnOffNew == 1){
sensePositionFunction();
headTurn();
}
if(headOnOffNew == 2){
}
}
else if(headOnOffNew != headOnOffOld){
headOnOffNew = headOnOffOld;
}
}
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//*********************************************************
//*********************************************************
//---------------------begin serialDoStuff -----------------
void serialDoStuff(){
if(Serial.available() > 0){
incomingHeadByte = Serial.read();
headOnOffAdjusted = incomingHeadByte - '0';
if(headOnOffAdjusted == 1){
Serial.flush();
// outgoingHeadByte = 1;
// Serial.write(outgoingHeadByte);
headOnOffOld = 1;
}
else if(headOnOffAdjusted == 2){
Serial.flush();
// outgoingHeadByte = 2;
// Serial.write(outgoingHeadByte);
headOnOffOld = 2;
}
}
}
//---------------------end serialDoStuff-----------------
//*********************************************************
//*********************************************************
//*********************************************************
//*********************************************************
// ++++++++++++++++++begin PIR function +++++++++++++++++++++++++++++++
void sensePositionFunction(){
for(int j=0;j<5;j++){ // do this for each PIR in the array
pirPin=pirNo[j];
pirStatus = digitalRead(pirPin);
if(pirStatus == HIGH){ // while cycling through the array if pirNo[j] is HIGH, do this
if(pirPrevLow[j]){ // and If pirNo[j] was previously low, do this
if(curPosPir != pirPin && pirPrevUsed[j] == 0){ // if pirNo[j] is HIGH and different than curPosPir, move to new position
curPosPir = pirPin; // keep current PIR
pirPrevUsed[j] = 1;
detectedPosition = pirPin;
// return detectedPosition;
}
pirPrevLow[j] = 0; // pir is now not low
}
}
else{
pirPrevLow[j] = 1; // pir is now low
pirPrevUsed[j] = 0;
}
} // end j number of pirs loop
}
//+++++++++++++++++++++++end PIR function +++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin headTurn -------------------
void headTurn() {
unsigned long currentMillis = millis();
// begin potservo section for potentiometer control
int valControl = detectedPosition; // reads the value of the potentiometer (value between 0 and 1023)
int valPosition = analogRead(potPositionPin);
valControl = map(valControl, 0, 1023, 0, 10); // first 0 refers to pot minimum, second is pot maximum,
valPosition = map(valPosition, 0, 1023, 0, 10); // third is minimum servo angle, fourth is maximum servo angle
// Serial.print("control ");
// Serial.println(valControl);
// Serial.print(" position ");
// Serial.println(valPosition);
if (valPosition > valControl)
{
// do Thing A
// turn on motor A forward
if (currentMillis - previousMillis > interval) {
previousMillis = currentMillis;
// set speed to __ out of possible range 80~255
analogWrite(enA, 100);
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
blinkSlow();
outgoingHeadByte = '<';
Serial.write(outgoingHeadByte);
}
}
else if (valPosition < valControl)
{
if (currentMillis - previousMillis > interval) {
previousMillis = currentMillis;
// do Thing B
// turn on motor A backward
// set speed to __ out of possible range 80~255
analogWrite(enA, 100);
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
blinkFast();
outgoingHeadByte = '>';
Serial.write(outgoingHeadByte);
}
}
else
{
previousMillis = currentMillis;
// do Thing C
// turn motor off
analogWrite(enA, 0);
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
}
}
//---------------------end headTurn--------------------
//*********************************************************
//*********************************************************
//---------------------begin blink slow-------------------
void blinkSlow(){
unsigned long currentMillis = millis();
const long interval1 = 500;
if(currentMillis - previousMillis >= interval1) {
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink slow-------------------
//---------------------begin blink fast -------------------
void blinkFast(){
unsigned long currentMillis = millis();
const long interval2 = 75;
if(currentMillis - previousMillis >= interval2){
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink fast -------------------
19102018 they are playing well together
C/C++I updated dc bias circuit schematic. The uno mouth code is more or less done for now. It receives commands and echos back. It gives led fast or slow flash without using delay commands to signify if uno is executing functions or waiting for an on command. The single sound input will be relatively easy to duplicate for a second input for mouth movements. The second dc bias circuit is also ready along with a line tap wire made from a headphone/aux cord. The serial functions in this sketch are the same as the head sketch.
/*
last edit Friday oct 19 2018
gravesunomouth
graves project
arduino Uno
Slave 1
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ (yellow signal / (red from speaker)
\ wire) /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
#include <Servo.h>
#include <SPI.h>
//---------------------begin declarations-----------------------
// for servo actions
Servo mouthServo;
// for gathering and mapping sound data
int soundPin=A5; // incoming lead from dc bias circuit
int soundDetected; // stores value from sound pin
int soundLevel; // converted values that only include 512-1024
int soundMap; // conversion from 512-1024 to 0-255
int servoMap; // conversion from 0-255 to 100-70
// communication
char incomingMouthByte; // for incoming serial data
char outgoingMouthByte; // for a bit more talkative serial data
int mouthOnOffNew; // stores mouth state from serial connection
int mouthOnOffOld; // stores mouth state from serial connection
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
// for blinking
const int ledPin = LED_BUILTIN;// the number of the LED pin
int ledState = LOW; // ledState used to set the LED
unsigned long previousMillis = 0; // will store last time LED was updated
//---------------------end declarations-----------------------
//*********************************************************
//*********************************************************
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
//---------------------begin void setup-----------------------
void setup(){
delay(5000);
Serial.begin(9600);
mouthServo.attach(6);
incomingMouthByte = 0; // for incoming serial data
outgoingMouthByte = 0; // for a little more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
pinMode(ledPin, OUTPUT);
}
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//---------------------end void setup-----------------------
//**********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//**********************************************************
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
//---------------------begin void loop-----------------------
void loop(){
serialDoStuff();
if(mouthOnOffNew == mouthOnOffOld){
if(mouthOnOffNew == 1){
blinkFast();
mouthDoStuff();
}
if(mouthOnOffNew == 2){
blinkSlow();
}
}
else if(mouthOnOffNew != mouthOnOffOld){
mouthOnOffNew = mouthOnOffOld;
}
}
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//---------------------end void loop-----------------------
//*********************************************************
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*********************************************************
//---------------------begin serialDoStuff -----------------
void serialDoStuff(){
if(Serial.available() > 0){
incomingMouthByte = Serial.read();
mouthOnOffAdjusted = incomingMouthByte - '0';
if(mouthOnOffAdjusted == 1){
Serial.flush();
outgoingMouthByte = 1;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 1;
}
else if(mouthOnOffAdjusted == 2){
Serial.flush();
outgoingMouthByte = 2;
Serial.write(outgoingMouthByte);
mouthOnOffOld = 2;
}
}
}
//---------------------end serialDoStuff-----------------
//*********************************************************
//*********************************************************
//---------------------begin mouthDoStuff -------------------
void mouthDoStuff(){
int soundDetected; // stores value from sound pin
int soundLevel; // converted values that only include 512-1024
int servoMap; // conversion from 0-255 to 100-70
soundDetected = analogRead(soundPin); // capture audio signal from dc bias circuit
if(soundDetected<=512){ // runs when negative amplitude detected
soundLevel = (512-soundDetected+512); // takes numbers below 512 and tacks em on top of 512 to create positive amplitudes
}
else{
soundLevel = soundDetected; // stores positive amplitudes
}
servoMap = map(soundLevel, 570, 610, 100, 50); // sound map 0-255 is mapped to truncated and inverted servo sweep 100-70
if(soundLevel < 700){
if(servoMap < 100){
mouthServo.write(servoMap);
Serial.print("soundLevel ");
Serial.println(soundLevel);
Serial.print(" servoMap ");
Serial.println(servoMap);
Serial.print(" soundDetected ");
Serial.println(soundDetected);
}
}
else{
mouthServo.write(95);
Serial.print("soundLevel ");
Serial.println(soundLevel);
Serial.print(" servoMap ");
Serial.println(servoMap);
Serial.print(" soundDetected ");
Serial.println(soundDetected);
}
// return soundLevel;
}
//// ++++++++++++++++++end mouthDoStuff +++++++++++++++++++++++++++++++++
//*********************************************************
//*********************************************************
//---------------------begin blink slow-------------------
void blinkSlow(){
unsigned long currentMillis = millis();
const long interval1 = 500;
if(currentMillis - previousMillis >= interval1) {
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink slow-------------------
//---------------------begin blink fast -------------------
void blinkFast(){
unsigned long currentMillis = millis();
const long interval2 = 75;
if(currentMillis - previousMillis >= interval2){
previousMillis = currentMillis;
if(ledState == LOW){
ledState = HIGH;
}
else{
ledState = LOW;
}
digitalWrite(ledPin, ledState);
}
}
//---------------------end blink fast -------------------
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
//*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-
Torture works!
C/C++And by that, I mean I tortured myself for days trying to get the mega to talk to the uno... then to get the uno to listen... then to get the uno to get the right message without changing it all around just to f with me... then to get the uno to talk back... then to get the mega to listen and understand. it all comes down to two major headaches. by trying to shortcut some intermediate programming, a whole of problems opens up for folks with crap knowledge so far like me. Timing a delay to wait for a response and getting the character data type to work around ascii tables makes my brain hurt
/*
last edit thursday oct 18 2018
working on serial coms
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//#include <Wire.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
char incomingMouthByte;
char outgoingMouthByte;
int mouthOnOffNew;
int mouthOnOffOld;
int mouthOnOffDifference;
int mouthOnOffAdjusted; // value adjusted by 48 for ascii
char incomingHeadByte;
char outgoingHeadByte;
int headOnOffNew;
int headOnOffOld;
int headOnOffDifference;
int headOnOffAdjusted; // value adjusted by 48 for ascii
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
Serial1.begin(9600);
Serial2.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 1;
outgoingHeadByte = 1; // for a bit more talkative serial data
headOnOffNew = 1; // stores head state from serial connection
headOnOffOld = 1; // stores head state from serial connection
incomingMouthByte = 0;
outgoingMouthByte = 0; // for a bit more talkative serial data
mouthOnOffNew = 1; // stores mouth state from serial connection
mouthOnOffOld = 1; // stores mouth state from serial connection
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){ // most regular pages
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){ // on off buttons
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
mouthUnoTxRx();
}
}
if(buttonGroup == 2){ // head buttons
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
headUnoTxRx();
}
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOffNew = 1; // on
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOffNew = 2; // off
Serial.print("mouthOnOffNew set to ");
Serial.println(mouthOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOffNew = 1; // on
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 2
if(164<p.y && p.y<240){
headOnOffNew = 2; // off
Serial.print("headOnOffNew set to ");
Serial.println(headOnOffNew);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
if(currentScreen == 122){
buttonGroup=1;
}
if(currentScreen == 222){
buttonGroup=1;
}
if(currentScreen == 23){
buttonGroup=2;
}
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
if(mouthOnOffNew != mouthOnOffOld){
Serial.println("starting txrx");
delay(10);
if(mouthOnOffNew == 1){
Serial.println("Starting tx");
delay(10);
Serial1.flush();
Serial1.write('1');
delay(10);
mouthOnOffOld = 1;
//begin rx
Serial.println("Starting rx");
delay(4000); // tweak delay to accomodate uno
// use a serial available after flush funtion while or for loop or interrrupt or somethin
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 1: ");
Serial.println(mouthOnOffAdjusted);
}
if(mouthOnOffNew == 2){
Serial.println("Starting tx");
delay(10);
Serial1.flush();
Serial1.write('2');
delay(20);
mouthOnOffOld = 2;
//begin rx
Serial.println("Starting rx");
delay(3000); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted should be 2: ");
Serial.println(mouthOnOffAdjusted);
}
else if(mouthOnOffAdjusted != 1 && mouthOnOffAdjusted != 2){
Serial.println("error reply wasn't like expected");
Serial.print("instead, the adjusted reply was");
delay(6000); // tweak delay to accomodate uno
incomingMouthByte = Serial1.read();
Serial1.flush();
mouthOnOffAdjusted = incomingMouthByte;
Serial.print("mouthOnOffAdjusted: ");
Serial.println(mouthOnOffAdjusted);
}
}
else if(mouthOnOffOld = mouthOnOffNew){
}
Serial.flush();
Serial1.flush();
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx --------------------------------------
void headUnoTxRx(){
if(headOnOffNew != headOnOffOld){
if(headOnOffNew == 1){
Serial2.write('1');
Serial.write('1');
headOnOffOld = 1;
delay(1000);
if(Serial2.available() > 0){
incomingHeadByte = Serial2.read();
headOnOffAdjusted = incomingHeadByte - 48;
if(headOnOffAdjusted == 1){
Serial2.write('1');
Serial.write('1');
}
}
}
if(headOnOffNew == 2){
Serial2.write('2');
Serial.write('2');
headOnOffOld = 2;
delay(1000);
if(Serial2.available() > 0){
incomingHeadByte = Serial2.read();
headOnOffAdjusted = incomingHeadByte - 48;
if(headOnOffAdjusted == 2){
Serial2.write('2');
Serial.write('2');
}
}
}
}
}
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(70, 100, 60, 30, BLACK);
Tft.drawNumber((buttonGroup), 70, 100, 3, GREEN);
Tft.fillRectangle(140, 100, 60, 30, BLACK);
Tft.drawNumber((mouthOnOffNew), 140, 100, 3, GREEN);
Tft.fillRectangle(160, 100, 60, 30, BLACK);
Tft.drawNumber((headOnOffNew), 160, 100, 3, GREEN);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Graves Head",0,5,3,BLUE);
Tft.drawString("Operations",10,50,3,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
// Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
do you expect me to talk?
C/C++No! I expect you to communicate over simple tx/rx ports like you already know how...even though I don't.
/*
last edit monday oct 15 2018 midnight
working on serial coms
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
#include <Wire.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
int incomingMouthByte;
int outgoingMouthByte;
int mouthOnOff;
int incomingHeadByte;
int outgoingHeadByte;
int headOnOff;
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 0;
incomingMouthByte = 0;
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){ // most regular pages
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){ // on off buttons
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
mouthUnoTxRx();
}
}
if(buttonGroup == 2){ // head buttons
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOff = 1; // on
Serial.print("mouthOnOff set to ");
Serial.println(mouthOnOff);
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOff = 0; // off
Serial.print("mouthOnOff set to ");
Serial.println(mouthOnOff);
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for head
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOff = 1; // on
}
//Button 2
if(164<p.y && p.y<240){
headOnOff = 0; // off
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
if(currentScreen == 122){
buttonGroup=1;
}
if(currentScreen == 222){
buttonGroup=1;
}
if(currentScreen == 23){
buttonGroup=2;
}
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
outgoingMouthByte = mouthOnOff;
if(Serial1){
Serial1.println(outgoingMouthByte); // send to serial connection
// incomingMouthByte = Serial1.read(); // read the incoming byte:
Serial.print("uno mouth wrote: ");
Serial.println(outgoingMouthByte);
}
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx --------------------------------------
// call slave1 arduino for data
void headUnoTxRx(){
outgoingHeadByte = headOnOff;
if(Serial2){
Serial2.println(outgoingHeadByte); // send to serial connection
incomingHeadByte = Serial2.read(); // read the incoming byte:
Serial.print("uno head received and wrote: ");
Serial.println(incomingHeadByte, DEC);
}
}
// call slave2 arduino for data
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(70, 160, 60, 30, BLACK);
Tft.drawNumber((mouthOnOff), 70, 160, 3, GREEN);
Tft.fillRectangle(140, 160, 60, 30, BLACK);
Tft.drawNumber((headOnOff), 140, 160, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Graves Head",0,5,3,BLUE);
Tft.drawString("Operations",10,50,3,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
// Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
button groups refined
C/C++this updated interface deals with changing button areas based on screen number
/*
last edit sunday oct 14 2018 midnight
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int buttonGroup; // sets kinds of buttons on screen
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
int incomingMouthByte;
int outgoingMouthByte;
int mouthOnOff;
int incomingHeadByte;
int outgoingHeadByte;
int headOnOff;
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
buttonGroup = 0;
incomingHeadByte = 0;
incomingMouthByte = 0;
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 15000; // 5000 = 5 seconds for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
if(buttonGroup == 0){
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 1){
tftButtonGroup1(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
if(buttonGroup == 2){
tftButtonGroup2(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
}
// serialDoStuff();
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOff = 1; // on
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOff = 0; // off
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for head
buttonPressed = 0;
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOff = 1; // on
}
//Button 2
if(164<p.y && p.y<240){
headOnOff = 0; // off
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
buttonGroup = 0;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
if(currentScreen == 122){
buttonGroup=1;
}
if(currentScreen == 222){
buttonGroup=1;
}
if(currentScreen == 23){
buttonGroup=2;
}
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
Serial1.write(mouthOnOff); // send a byte with the value 45
if(Serial1.available() > 0){
incomingMouthByte = Serial1.read(); // read the incoming byte:
}
}
//--------------------------end mouthUnoTxRx --------------------------------------
//-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----*-----
//--------------------------begin headUnoTxRx --------------------------------------
// call slave1 arduino for data
void headUnoTxRx(){
Serial2.write(headOnOff); // send a byte with the value 45
if(Serial2.available() > 0){
incomingHeadByte = Serial2.read(); // read the incoming byte:
}
}
// call slave2 arduino for data
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 225, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 225, 3, GREEN);
Tft.fillRectangle(180, 225, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 225, 3, GREEN);
Tft.fillRectangle(100, 225, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 100, 225, 3, GREEN);
Tft.fillRectangle(70, 160, 60, 30, BLACK);
Tft.drawNumber((mouthOnOff), 70, 160, 3, GREEN);
Tft.fillRectangle(140, 160, 60, 30, BLACK);
Tft.drawNumber((headOnOff), 140, 160, 3, GREEN);
Tft.fillRectangle(120, 290, 60, 30, BLACK);
Tft.drawNumber((currentScreen), 120, 290, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Graves Head",0,5,3,BLUE);
Tft.drawString("Operations",10,50,3,YELLOW);
Tft.drawString("On", 120, 130, 3, GREEN);
Tft.drawString("Off", 115, 195, 3, RED);
// Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0422 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 422){
// " "
Tft.drawString("Command Sent",0,5,3,BLUE);
Tft.drawString("Press Back to",0,55,3,YELLOW);
Tft.drawString("Contiue", 50,105, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0422 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
/*
last edit sun oct 14 2018 later in the day
gravesmegamaster
graves project
arduino MEGA 2650
Master
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
int incomingMouthByte;
int outgoingMouthByte;
int mouthOnOff;
int incomingHeadByte;
int outgoingHeadByte;
int headOnOff;
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(9600);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
incomingHeadByte = 0;
incomingMouthByte = 0;
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 5000; // for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout( ); // check time and turn off backlight
tftButtonGroup0(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
// serialDoStuff();
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
//--------------------------begin tftButtonGroup0----------------------------------
int tftButtonGroup0(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
//Button 5
if(250<p.x && p.x<275){
if(0<p.y && p.y<75){
buttonPressed = 5;
}
}
}//void tftButtonGroup0
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//--------------------------end tftButtonGroup0 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
//--------------------------begin tftButtonGroup1----------------------------------
int tftButtonGroup1(){ // for mouth
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
mouthOnOff = 1; // on
}
//Button 2
if(164<p.y && p.y<240){
mouthOnOff = 0; // off
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
// }
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup1
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//--------------------------end tftButtonGroup1 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
//--------------------------begin tftButtonGroup2----------------------------------
int tftButtonGroup2(){ // for head
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
headOnOff = 1; // on
}
//Button 2
if(164<p.y && p.y<240){
headOnOff = 0; // off
}
//Button 3
// if(240<p.y && p.y<310){
// buttonPressed = 3;
// }
// }
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftButtonGroup2
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//--------------------------end tftButtonGroup2 code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin mouthUnoTxRx----------------------------------
void mouthUnoTxRx(){
//mouthOnOff code?
Serial1.write(1); // send a byte with the value 45
if (Serial1.available() > 0) {
// read the incoming byte:
incomingMouthByte = Serial1.read();
}
}
//--------------------------end mouthUnoTxRx --------------------------------------
//--------------------------begin headUnoTxRx --------------------------------------
// call slave1 arduino for data
//headOnOff code?
void headUnoTxRx(){
Serial2.write(1); // send a byte with the value 45
if (Serial2.available() > 0) {
// read the incoming byte:
incomingHeadByte = Serial2.read();
}
}
// call slave2 arduino for data
//--------------------------end headUnoTxRx --------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 160, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 160, 3, GREEN);
Tft.fillRectangle(180, 160, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 160, 3, GREEN);
Tft.fillRectangle(80, 160, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 80, 160, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin mouth on off function --------------------------
//-------------------------- end mouth on off function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin head on off function --------------------------
//-------------------------- end head on off function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Manual Point",10,50,4,YELLOW);
Tft.drawString("Slider", 80,130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
13102018 - Bodmer to the rescue!
C/C++Big shout out to Bodmer for helping identify my spotty understanding of data types. I had wrongly used int to store a millis() value and the prog would crash after a minute or two. changed them to unsigned longs and it chooches along flawlessly now! I was going to rewrite menus, but now I think I'll wait to establish communication from the mega to the dos uno's. I'll figure out what data I can pass back and forth before the menu rewrite. Thanks again, Bodmer!
/*
last edit sun oct 13 2018
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int buttonPressed; // stores which button was pressed
unsigned long newButtonTime; // debounce
unsigned long oldButtonTime; // debounce
unsigned long buttonTimeDifference; // debounce
unsigned long debounceTime; // debounce
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
unsigned long backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
unsigned long backlightTimeDifference;
unsigned long newBacklightTime;
unsigned long oldBacklightTime;
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(115200);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 5000; // for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
tftDetect(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
int tftDetect(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
//Button 5
if(250<p.x && p.x<275){
if(0<p.y && p.y<75){
buttonPressed = 5;
}
}
}//void tftDetect
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin arduino master data fetch calls----------------------------------
// call slave1 arduino for data
// call slave2 arduino for data
//--------------------------end arduino master data fetch calls------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 160, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 160, 3, GREEN);
Tft.fillRectangle(180, 160, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 160, 3, GREEN);
Tft.fillRectangle(80, 160, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 80, 160, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave1 data fetch function --------------------------
//-------------------------- end slave1 data fetch function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave2 data fetch function --------------------------
//-------------------------- end slave2 data fetch function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Manual Point",10,50,4,YELLOW);
Tft.drawString("Slider", 80,130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
12102018 later in the day
C/C++updated code again. this time with a back light time out. can't figure out why the screen paging program is failing after a minute or two while the back light timer continues. gonna post the code and ask for help.
/*
last edit sun oct 12 2018 later in the day
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int buttonPressed; // stores which button was pressed
int newButtonTime; // debounce
int oldButtonTime; // debounce
int buttonTimeDifference; // debounce
int debounceTime; // debounce
int currentScreen; // identifies current screen by adding x y and z
int x; // for ones
int y; // for tens
int z; // for hundreds
int zMax; // maximum pressure to detect
int zMin; // minimum pressure to detect
int backlightTimer; // for backlight timeout
int backLightState; // status of backlight 1 for on and 0 for off
int backlightTimeDifference;
int newBacklightTime;
int oldBacklightTime;
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(115200);
oldButtonTime = millis();
newButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
backLightState = 1;
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
////////////////////////////////
//// manually set variables ////
////////////////////////////////
debounceTime = 1000; // button debounce in milliseconds
zMax = 460; // maximum pressure to detect
zMin = 100; // minimum pressure to detect
backlightTimer = 5000; // for backlight timeout
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftBacklightTimeout(); // check time and turn off backlight
tftDetect(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftBacklightTimeout----------------------------------
void tftBacklightTimeout(){
newBacklightTime = millis();
if(backLightState = 0){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer > backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
}
}
if(backLightState = 1){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
if(backlightTimer < backlightTimeDifference){
backlightTimeDifference = newBacklightTime - oldBacklightTime;
TFT_BL_OFF; // turn off the background light
backLightState = 0;
oldBacklightTime = millis();
}
}
}
//--------------------------end tftBacklightTimeout----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
int tftDetect(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
TFT_BL_ON; // turn on the background light
backLightState = 1;
oldBacklightTime = millis();
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
//Button 5
if(250<p.x && p.x<275){
if(0<p.y && p.y<75){
buttonPressed = 5;
}
}
}//void tftDetect
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
if(buttonPressed == 5){
x = 0;
y = 0;
z = 0;
Serial.println("xyz all 0");
}
}//y>0
}//x>0
currentScreen = z+y+x;
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin arduino master data fetch calls----------------------------------
// call slave1 arduino for data
// call slave2 arduino for data
//--------------------------end arduino master data fetch calls------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 160, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 160, 3, GREEN);
Tft.fillRectangle(180, 160, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 160, 3, GREEN);
Tft.fillRectangle(80, 160, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 80, 160, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave1 data fetch function --------------------------
//-------------------------- end slave1 data fetch function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave2 data fetch function --------------------------
//-------------------------- end slave2 data fetch function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
********
updates needed
********
1 ditch the contrast
2 ditch the brightness
3 setup screen timeout
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Manual Point",10,50,4,YELLOW);
Tft.drawString("Slider", 80,130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
draft 12102018
C/C++latest update
finished sorting out page navigation
need to clean up colors and word positions
will fill in data fetch later
finished sorting out page navigation
need to clean up colors and word positions
will fill in data fetch later
/*
last edit sun oct 12 2018
coded from scratch by wylie jones
wyliejones@gmail.com
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//end notes
//end notes
//end notes
//end notes
//end notes
//end notes
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//begin declarations
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
int buttonPressed; // stores which button was pressed
int newButtonTime; // debounce
int oldButtonTime; // debounce
int buttonTimeDifference; // debounce
int debounceTime; // debounce
int currentScreen; // identifies current screen by adding x y and z
int zMax = 460; // maximum pressure to detect
int zMin = 100; // minimum pressure to detect
int x; // for ones
int y; // for tens
int z; // for hundreds
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//end declarations
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
Serial.begin(115200);
oldButtonTime = millis();
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
x = 0;
y = 0;
z = 0;
buttonPressed = 4;
debounceTime = 1000; // button debounce in milliseconds
currentScreen = 0;
callScreen();
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
tftDetect(); // gather information from touch screen touches
buttonTimeDifference = newButtonTime - oldButtonTime;
if(debounceTime < buttonTimeDifference){
whatScreen();
callScreen();
oldButtonTime = newButtonTime;
showStuff(); // serial prints
tftDisplay(); // display x y coordinates on tft display
}
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
/////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
//--------------------------begin tftDetect----------------------------------
int tftDetect(){
Point p = ts.getPoint();
if(zMin<p.z && p.z<zMax){
newButtonTime = millis();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
//Button 1
if(75<p.x && p.x<140){
if(100<p.y && p.y<164){
buttonPressed = 1;
}
//Button 2
if(164<p.y && p.y<240){
buttonPressed = 2;
}
//Button 3
if(240<p.y && p.y<310){
buttonPressed = 3;
}
}
}
//Button 4
if(10<p.x && p.x<75){
if(100<p.y && p.y<240){
buttonPressed = 4;
}
}
}//void tftDetect
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//--------------------------end tftDetect code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
//-------------------------- begin whatScreen function -------------------------
int whatScreen(){
if(x == 0){
if(buttonPressed == 1){
x = x + 1;
Serial.println("x + 1");
}
if(buttonPressed == 2){
x = x + 2;
Serial.println("x + 2");
}
if(buttonPressed == 3){
x = x + 3;
Serial.println("x + 3");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
}
else if(x >> 0){
if(y == 0){
if(buttonPressed == 1){
y = y + 10;
Serial.println("y + 10");
}
if(buttonPressed == 2){
y = y + 20;
Serial.println("y + 20");
}
if(buttonPressed == 3){
y = y + 30;
Serial.println("y + 30");
}
if(buttonPressed == 4){
x = 0;
Serial.println("x = 0");
}
}
else if (y >> 0){
if(buttonPressed == 1){
z = z + 100;
Serial.println("z + 100");
}
if(buttonPressed == 2){
z = z + 200;
Serial.println("z + 200");
}
if(buttonPressed == 3){
z = z + 300;
Serial.println("z + 300");
}
if(buttonPressed == 4){
if(z == 0){
y = 0;
Serial.println("y = 0");
}
else if(z >> 0){
z = 0;
Serial.println("z = 0");
}
}
}//y>0
}//x>0
currentScreen = z+y+x;
}
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//-------------------------- end whatScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin arduino master data fetch calls----------------------------------
// call slave1 arduino for data
// call slave2 arduino for data
//--------------------------end arduino master data fetch calls------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin tftDisplay code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
Tft.fillRectangle(0, 160, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 160, 3, GREEN);
Tft.fillRectangle(180, 160, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 160, 3, GREEN);
Tft.fillRectangle(80, 160, 60, 30, BLACK);
Tft.drawNumber((buttonPressed), 80, 160, 3, GREEN);
}// void tftDisplay
//--------------------------end tftDisplay code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave1 data fetch function --------------------------
//-------------------------- end slave1 data fetch function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave2 data fetch function --------------------------
//-------------------------- end slave2 data fetch function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin serial display function --------------------------
void showStuff(){
Serial.println("------/ last update /-------");
// Serial.println("------begin display-------");
Serial.print("buttonPressed ");
Serial.println(buttonPressed);
Serial.print("x ");
Serial.println(x);
Serial.print("y ");
Serial.println(y);
Serial.print("z ");
Serial.println(z);
Serial.print("currentScreen ");
Serial.println(currentScreen);
// Serial.println("-------end display--------");
Serial.println("");
Serial.println("");
Serial.println("");
}
//-------------------------- end serial display function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
screen 0000 A)Home
screen 0001 a)settings
screen 0011 -brightness
screen 0011 -contrast
screen 0011 -screen lock time out
screen 0001 b)sensor monitors
screen 0021 -pir
screen 0121 *report pir detection
screen 0021 -sound
screen 0221 *sense alexa sound
screen 0221 *sense graves sound
screen 0221 *report mouth servo position
screen 0221 *adjust sensitivity?
screen 0021 -head potentiometer
screen 0021 -bluetooth
screen 0001 c)bluetooth
screen 0031 -on
screen 0031 -off
screen 0031 -monitor
screen 0000 B)Mouth Control
screen 0002 a)test button
screen 0012 -open
screen 0012 -close
screen 0012 -push button chicken switch
screen 0002 b)sound sensors on/off
screen 0022 -alexa sense
screen 0122 *on
screen 0122 *off
screen 0122 *monitor sensor
screen 0022 -graves sense
screen 0222 *on
screen 0222 *off
screen 0222 *monitor sensors
screen 0002 c)sound sensor status
screen 0032 -alexa
screen 0032 -graves
screen 0000 C)Head Control
screen 0003 a)pir on/off
screen 0013 -on
screen 0013 -off
screen 0013 -sensor
screen 0003 b)manual point
screen 0023 -slider
*/
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//<*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*><*>
//****************************************************************************************************
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
//-------------------------- begin callScreen function -------------------------
int callScreen(){
Tft.fillRectangle(0, 0, 240, 320, BLACK);
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0000 master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 0){
// " "
Tft.drawString("GRAVES",0,5,4, GREEN);
Tft.drawString("INTERFACE",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0000 master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0001 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 1){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("FUNCTIONS",00,50,4,BLUE);
Tft.drawString("Settings", 10,130, 3, BLUE);
Tft.drawString("Sensors", 20, 195, 3, BLUE);
Tft.drawString("Bluetooth", 00, 260, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0001 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0011 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 11){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SETTINGS",10,50,4,YELLOW);
Tft.drawString("Brightness", 80,130, 3, BLUE);
Tft.drawString("Contrast", 05, 195, 3, GREEN);
Tft.drawString("Timeout", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0011 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0021 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 21){
// " "
Tft.drawString("MEGA 2560",00,5,4,BLUE);
Tft.drawString("SENSORS",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Sound", 05, 195, 3, GREEN);
Tft.drawString("Head Position", 10, 260, 3, GREEN);
Tft.drawString("Bluetooth", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0021 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0121 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 121){
// " "
Tft.drawString("PIR",0,5,4,BLUE);
Tft.drawString("ARRAY",10,50,4,YELLOW);
Tft.drawString("PIR sensor #", 80, 60, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 85, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 105, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("PIR sensor #", 80, 130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0121 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0221 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 221){
// " "
Tft.drawString("SOUND",0,5,4,BLUE);
Tft.drawString("Sense Alexa",10,50,4,YELLOW);
Tft.drawString("Sense Graves", 80,130, 3, BLUE);
Tft.drawString("Jaw position", 05, 195, 3, GREEN);
Tft.drawString("Jaw Settings", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0221 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0031 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 31){
// " "
Tft.drawString("Bluetooth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0031 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0002 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 2){
// " "
Tft.drawString("Mouth",0,5,4,BLUE);
Tft.drawString("Control",10,50,4,YELLOW);
Tft.drawString("Test Jaw", 80,130, 3, BLUE);
Tft.drawString("Sensor on/off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0002 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0012 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 12){
// " "
Tft.drawString("Jaw Test",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("Open", 80,130, 3, BLUE);
Tft.drawString("Close", 05, 195, 3, GREEN);
Tft.drawString("Chickn Switch", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0012 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0022 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 22){
// " "
Tft.drawString("Sound",0,5,4,BLUE);
Tft.drawString("Sensors",10,50,4,YELLOW);
Tft.drawString("Alexa Sense", 80,130, 3, BLUE);
Tft.drawString("Graves Sense", 05, 195, 3, GREEN);
Tft.drawString("Monitor", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0022 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0122 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 122){
// " "
Tft.drawString("Alexa Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0122 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0222 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 222){
// " "
Tft.drawString("Graves Sense",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Monitor Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0222 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0032 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 32){
// " "
Tft.drawString("Sound Sense",0,5,4,BLUE);
Tft.drawString("Monitors",10,50,4,YELLOW);
Tft.drawString("Alexa", 80,130, 3, BLUE);
Tft.drawString("Graves", 05, 195, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0032 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0003 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 3){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("PIR Array", 80,130, 3, BLUE);
Tft.drawString("Motor On/Off", 05, 195, 3, GREEN);
Tft.drawString("Manual Point", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0003 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0013 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 13){
// " "
Tft.drawString("PIR Control",0,5,4,BLUE);
Tft.drawString("Operations",10,50,4,YELLOW);
Tft.drawString("On", 80,130, 3, BLUE);
Tft.drawString("Off", 05, 195, 3, GREEN);
Tft.drawString("Current Sense", 10, 260, 3, GREEN);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0013 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 0023 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
if(currentScreen == 23){
// " "
Tft.drawString("Head Control",0,5,4,BLUE);
Tft.drawString("Manual Point",10,50,4,YELLOW);
Tft.drawString("Slider", 80,130, 3, BLUE);
Tft.drawString("<", 0, 160, 3, GREEN); // back button
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 0023 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> begin screen lost <><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
// else if(currentScreen){
// // " "
// Tft.drawString("Screen not",0,5,3,BLUE);
// Tft.drawString("found. Please",40,50,3,YELLOW);
// Tft.drawString("use the back", 80,130, 3, BLUE);
// Tft.drawString("button to", 05, 195, 3, GREEN);
// Tft.drawString("return to fun", 10, 260, 3, GREEN);
// Tft.drawString("<", 0, 160, 3, GREEN); // back button
//}
////<><><><><><><><><><><><><><><><><><><><><><><><>
////<><><><><><><><> end screen lost <><><><><><><><><>
////<><><><><><><><><><><><><><><><><><><><><><><><>
}// callScreen
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//-------------------------- end callScreen function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
/*
last edit sun oct 7 2018
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
//#include <TFT.h>
//#include <Servo.h>
//#include <stdint.h>
//#include <SD.h>
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//const int PIN_SD_CS = 4; // pin of sd card
TouchScreen ts = TouchScreen(XP, YP, XM, YM);
//*********************************************************
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
// need timer to track screen timeout
// set screen to on and let timer run to turn off
Tft.TFTinit(); // init TFT library
TFT_BL_ON; // turn on the background light
screenMaster(); //calls function to draw title and menu items
} //void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
//tftDetect(); // gather information from touch screen touches
tftDisplay(); // display x y coordinates on tft display
} //void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
/////////////////////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////// ///////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
//////////////////////////////////////// ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin TFT input code----------------------------------
void tftDetect(){
// a point object holds x y and z coordinates
Point p = ts.getPoint();
if(100<p.z && p.z<460){ // max and min z variable later? acts as debounce
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
}//if
}//void tftDetect
//--------------------------end TFT intput code----------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin arduino master data fetch calls----------------------------------
// call slave1 arduino for data
// call slave2 arduino for data
//--------------------------end arduino master data fetch calls------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin TFT output code----------------------------------
void tftDisplay(){
Point p = ts.getPoint();
p.x = map(p.x, TS_MINX, TS_MAXX, 0, 240);
p.y = map(p.y, TS_MINY, TS_MAXY, 0, 320);
// p.z = map(p.z, 0, 600, 100, 0);
if(100<p.z && p.z<460){ // max and min z variable later? acts as debounce
Tft.fillRectangle(0, 160, 60, 30, BLACK);
Tft.drawNumber((p.x), 0, 160, 3, GREEN);
Tft.fillRectangle(180, 160, 60, 30, BLACK);
Tft.drawNumber((p.y), 180, 160, 3, GREEN);
}//if
}// void tftDisplay
//--------------------------end TFT output code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave1 data fetch function --------------------------
//-------------------------- end slave1 data fetch function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave2 data fetch function --------------------------
//-------------------------- end slave2 data fetch function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
/*
background screen file names on sd card
pipboybg.bmp
pipboylocked.bmp
tft2buttons.bmp
tft3buttons.bmp
*/
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
text size 3 can fit 13 characters
screen 1 A)master
screen 2 a)settings
screen 2 -brightness
screen 2 -contrast
screen 2 -screen lock time out
screen 3 b)sensor monitors
screen 3 -pir
screen 4 *report pir detection
screen 4 *report head servo position
screen 3 -sound
screen 5 *sense alexa sound
screen 5 *sense graves sound
screen 5 *report mouth servo position
screen 5 *adjust sensitivity?
screen 3 c)bluetooth
screen 6 -on
screen 6 -off
screen 6 -monitor
screen 7 B)slave1 mouth servo
screen 7 a)test button
screen 8 -open
screen 8 -close
screen 8 -push button chicken switch
screen 7 b)sound sensors on/off
screen 9 -alexa sense
screen 10 *on
screen 10 *off
screen 10 *monitor sensor
screen 9 -graves sense
screen 11 *on
screen 11 *off
screen 11 *monitor sensors
screen 7 c)sound sensor status
screen 12 -alexa
screen 12 -graves
screen 13 C)slave2 head servo
screen 14 a)pir on/off
screen 15 -on
screen 15 -off
screen 14 b)manual point
screen 16 -slider
*/
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen master <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
void screenMaster(){
// " "
Tft.drawString("Undertaker",0,5,4,GREEN);
Tft.drawString("Interface",10,50,4,GREEN);
Tft.drawString("Home", 80,130, 3, GREEN);
Tft.drawString("Mouth Control", 05, 195, 3, GREEN);
Tft.drawString("Head Control", 10, 260, 3, GREEN);
}
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen master <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 1 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 1 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 2 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 2 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 3 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 3 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 4 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 4 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 5 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 5 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 6 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 6 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 7 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 7 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 8 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 8 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 9 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 9 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 10 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 10 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 11 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 11 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> begin screen 12 <><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//<><><><><><><><> end screen 12 <><><><><><><><><>
//<><><><><><><><><><><><><><><><><><><><><><><><>
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
/*
Graves multi arduino build 10/2018
Arduino mega 2560 with 240/320 tft and micro sd card (for storing screen backgrounds)
two arduino uno's
slave1 arduino uno to sense sound from graves and echo dot to control mouth
slave2 arduino uno to control passive sensor array and head servo
sound is sensed with a simple DC bias circuit to capture line level input to be used as a digital signal
analogue pin speaker +
\ / (red from speaker)
\ /
\ /
*--------[=]-----------*---------[=]----------*
| resistor \ resistor |
| \ |
|(red with white) \ |to arduino ground (black with white)
to arduino 5v+ speaker -
(black from speaker)
*/
#include <TFTv2.h>
#include <SPI.h>
#include <SeeedTouchScreen.h>
//*********************************************************
//*********************************************************
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
// begin void setup
void setup() {
// need timer to track screen timeout
// set screen to on and let timer run to turn off
} // void setup()
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
// end void setup
//*********************************************************
//*********************************************************
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
// begin void loop
void loop() {
// look for interrupt
//
} // void loop()
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
// end of void loop
/////////////////////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////// ///////////////////////////////////////////////////
///////////////////////////////////////// functions ///////////////////////////////////////////////////
//////////////////////////////////////// ///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin arduino master data fetch calls----------------------------------
// call slave1 arduino for data
// call slave2 arduino for data
//--------------------------end arduino master data fetch calls------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//--------------------------begin TFT output code----------------------------------
// int displaySensorLogs;
// displaySensorLogs = tftOutputFunction();
//--------------------------end TFT output code------------------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave1 data fetch function --------------------------
//-------------------------- end slave1 data fetch function --------------------------
//****************************************************************************************************
//****************************************************************************************************
//-------------------------- begin slave2 data fetch function --------------------------
//-------------------------- end slave2 data fetch function -------------------------
//****************************************************************************************************
//****************************************************************************************************
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ screens \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//****************************************************************************************************
//****************************************************************************************************
//--------------------------- Primary screen maps --------------------------------
/*
A)master
a)settings
-brightness
-contrast
-screen lock time out
b)sensor monitors
-pir
*report pir detection
*report head servo position
-sound
*sense alexa sound
*sense graves sound
*report mouth servo position
*adjust sensitivity?
c)bluetooth
-on
-off
-monitor
B)slave1 mouth servo
a)test button
-open
-close
-push button chicken switch
b)sound sensors on/off
-alexa sense
*on
*off
*monitor sensor
-graves sense
*on
*off
*monitor sensors
c)sound sensor status
-alexa
-graves
C)slave2 head servo
a)pir on/off
-on
-off
b)manual point
*/
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
//****************************************************************************************************
// fin
Comments
Please log in or sign up to comment.